DJMaxus Posted September 21, 2010 Author Share Posted September 21, 2010 This is a tutorial for Eclipse Origins v.2.0.0\. It will allow you to combine two items into one item as long as you have the proper tool equipped and the recipe for the item. I have finally gotten around to updating it to work with EOv2 with no problems. Just re-follow this tutorial here in the OP and you will be all good. **I recommend you expand it and modify it to fit your game.***I've included **a new frmEditor_Item** that is ready for use with this tutorial._**How It Works:**_You will be creating a new item type called Recipe, this item will contain four variables, the two items needed, the resulting item, and the tool needed to use this recipe. The tool needed can be a hammer, mortar and pestle, magic staff, whatever. When creating a weapon, a new option for it will also be added that determines what kind of tool the item is, such as a hammer. When you use a recipe item, and you have the required items, as well as have the proper tool equipped to your character, you will successfully create the item and the recipe will disappear. (Or you can make it to where the recipe does not disappear with a simple edit)_**The Code:**_**Server Side**In **modTypes**Find : **Private Type ItemRec**Add this to the bottom of **Private Type ItemRec** (before **End Type**)```  Tool As Long  ToolReq As Long```In **modConstants**Find:```Public Const ITEM_TYPE_SPELL As Byte = 8```Add this below:```Public Const ITEM_TYPE_RECIPE As Byte = 9```In **modPlayer**Find the **UseItem** sub.Near the top of the sub, find:```Dim n As Long, i As Long, tempItem As Long, x As Long, y As Long, itemnum As Long```Add this below:```Dim Item1 As LongDim Item2 As LongDim Result As Long```And add the following before the last **End Select** (Still in the same sub)```Case ITEM_TYPE_RECIPE        ' Get the recipe information        Item1 = Item(GetPlayerInvItemNum(Index, InvNum)).Data1        Item2 = Item(GetPlayerInvItemNum(Index, InvNum)).Data2        Result = Item(GetPlayerInvItemNum(Index, InvNum)).Data3        ' Perform Recipe checks        If Item1 <= 0 Then          Call PlayerMsg(Index, "This is an incomplete recipe...", White)          Exit Sub        End If        If Item2 <= 0 Then          Call PlayerMsg(Index, "This is an incomplete recipe...", White)          Exit Sub        End If        If Result <= 0 Then          Call PlayerMsg(Index, "This is an incomplete recipe...", White)          Exit Sub        End If        If GetPlayerEquipment(Index, Weapon) <= 0 Then          Call PlayerMsg(Index, "You don't have the proper tool equipped!", White)          Exit Sub        End If        If Item(GetPlayerEquipment(Index, Weapon)).Tool = Item(GetPlayerInvItemNum(Index, InvNum)).ToolReq Then          ' Give the resulting item            If HasItem(Index, Item1) Then              If HasItem(Index, Item2) Then                Call TakeInvItem(Index, Item1, 1)                Call TakeInvItem(Index, Item2, 1)                Call GiveInvItem(Index, Result, 1)                Call TakeInvItem(Index, GetPlayerInvItemNum(Index, InvNum), 0)                Call PlayerMsg(Index, "You have successfully created " & Trim(Item(Result).Name) & ".", White)              Else                Call PlayerMsg(Index, "You do not have all of the ingredients.", White)                Exit Sub              End If            Else              Call PlayerMsg(Index, "You do not have all of the ingredients.", White)              Exit Sub            End If          Else            Call PlayerMsg(Index, "This is not the tool used in this recipe.", White)            Exit Sub          End If```**Client Side**In **modTypes**Find : **Private Type ItemRec**Add this to the bottom of **Private Type ItemRec** (before **End Type**)```  Tool As Long  ToolReq As Long```In **modConstants**Find:```Public Const ITEM_TYPE_SPELL As Byte = 8```Add this below:```Public Const ITEM_TYPE_RECIPE As Byte = 9```In **modGameEditors**Find:```If (frmEditor_Item.cmbType.ListIndex = ITEM_TYPE_SPELL) Then      frmEditor_Item.fraSpell.Visible = True      frmEditor_Item.scrlSpell.Value = .Data1    Else      frmEditor_Item.fraSpell.Visible = False    End If```Add this below:```If (frmEditor_Item.cmbType.ListIndex = ITEM_TYPE_RECIPE) Then      frmEditor_Item.fraRecipe.Visible = True      frmEditor_Item.scrlItem1.Value = .Data1      frmEditor_Item.scrlItem2.Value = .Data2      frmEditor_Item.scrlResult.Value = .Data3      frmEditor_Item.cmbCToolReq.ListIndex = .ToolReq    Else      frmEditor_Item.fraRecipe.Visible = False    End IffrmEditor_Item.cmbCTool.ListIndex = .Tool```In **modGameLogic**Find the **UpdateDescWindow** subNear the top of the sub, find:```Dim Name As String```Add this below:```Dim Item1 As LongDim Item2 As LongDim Tool As String```In the same sub, find this:```' set z-order  frmMain.picItemDesc.ZOrder (0)```Underneath it add this:```Item1 = Item(itemnum).Data1  Item2 = Item(itemnum).Data2  Select Case Item(itemnum).ToolReq    Case 0 'None      Tool = "None"    Case 1 'Hammer      Tool = "Hammer"    Case 2 'Mortar & Pestle      Tool = "Mortar and Pestle"  End Select```Find this:```' set captions    .lblItemName.Caption = Name    .lblItemDesc.Caption = Trim$(Item(itemnum).Desc)```Underneath it add this:```If Item(itemnum).Type = ITEM_TYPE_RECIPE Then      .lblItemDesc.Caption = "This recipe calls for a " & Trim$(Item(Item1).Name) & " and " & Trim$(Item(Item2).Name) & ". Tool required: " & Trim$(Tool)    End If```If you decide to use the frmEditor_Item I have included with this topic, you do not have to follow the steps below and are done with the tutorial.**_Form Work:_**Open up **frmEditor_Item**Add the following items to it: (You may have to extend the form and make room)1\. Frame, call it fraRecipe (Visible should be set to false)2\. ComboBox, call it cmbCToolThe following should be placed within fraRecipe3\. Label, call it lblItem14\. Label, call it lblItem25\. Label, call it lblResult6\. Scroll Bar, call it scrlItem17\. Scroll Bar, call it scrlItem28\. Scroll Bar, call it scrlResult9\. ComboBox, call it cmbCToolReqDouble click scrlItem1 and paste this:```If scrlItem1.Value > 0 Then    lblItem1.Caption = "Item: " & Trim$(Item(scrlItem1.Value).Name)  Else    lblItem1.Caption = "Item: None"  End If  Item(EditorIndex).Data1 = scrlItem1.Value```Double click scrlItem2 and paste this:```If scrlItem2.Value > 0 Then    lblItem2.Caption = "Item: " & Trim$(Item(scrlItem2.Value).Name)  Else    lblItem2.Caption = "Item: None"  End If  Item(EditorIndex).Data2 = scrlItem2.Value```Double click scrlResult and paste this:```If scrlResult.Value > 0 Then    lblResult.Caption = "Result: " & Trim$(Item(scrlResult.Value).Name)  Else    lblResult.Caption = "Result: None"  End If  Item(EditorIndex).Data3 = scrlResult.Value```Double click cmbCToolReq, make the whole sub look like this:```Private Sub cmbCToolReq_Click()  If EditorIndex = 0 Or EditorIndex > MAX_ITEMS Then Exit Sub  Item(EditorIndex).ToolReq = cmbCToolReq.ListIndexEnd Sub```Double click cmbCTool, make the whole sub look like this:```Private Sub cmbCTool_Click()  If EditorIndex = 0 Or EditorIndex > MAX_ITEMS Then Exit Sub  Item(EditorIndex).Tool = cmbCTool.ListIndexEnd Sub```In cmbCTool and cmbCToolReq, go to their properties, find the "List" option, click it and add the following into it:```NoneHammerMortar & Pestle```In cmbType, go to its properties, find the "List" options, click it and add this to the bottom:```Recipe```Double click cmbType in the ItemEditorFind this:```If (cmbType.ListIndex = ITEM_TYPE_SPELL) Then    fraSpell.Visible = True  Else    fraSpell.Visible = False  End If```Underneath it add this:```If (cmbType.ListIndex = ITEM_TYPE_RECIPE) Then    fraRecipe.Visible = True  Else    fraRecipe.Visible = False  End If```Once you do this, you should be all finished with this tutorial. Hope this helps you further your project. I may be missing something in this tutorial because I made this in a hurry, but if so I will try to fix the mistakes/things I've missed. Enjoy the tut. Link to comment Share on other sites More sharing options...
Dzastin Posted September 21, 2010 Share Posted September 21, 2010 When I inserted the code in my error displays:[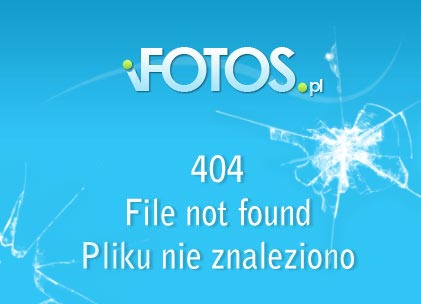](http://ifotos.pl/zobacz/error_srhwse.JPG/)>! Case ITEM_TYPE_RECIPE        ' Get the recipe information        Item1 = Item(GetPlayerInvItemNum(Index, InvNum)).Data1        Item2 = Item(GetPlayerInvItemNum(Index, InvNum)).Data2        Result = Item(GetPlayerInvItemNum(Index, InvNum)).Data3        ' Perform Recipe checks        If Item1 <= 0 Then          Call PlayerMsg(Index, "This is an incomplete recipe…", White)          Exit Sub        End If        If Item2 <= 0 Then          Call PlayerMsg(Index, "This is an incomplete recipe...", White)          Exit Sub        End If        If Result <= 0 Then          Call PlayerMsg(Index, "This is an incomplete recipe...", White)          Exit Sub        End If        If GetPlayerEquipment(Index, Weapon) <= 0 Then          Call PlayerMsg(Index, "You don't have the proper tool equipped!", White)          Exit Sub        End If        If Item(GetPlayerEquipment(Index, Weapon)).Tool = Item(GetPlayerInvItemNum(Index, InvNum)).ToolReq Then          ' Give the resulting item            If HasItem(Index, Item1) Then              If HasItem(Index, Item2) Then                Call TakeInvItem(Index, Item1, 1)                Call TakeInvItem(Index, Item2, 1)                Call GiveInvItem(Index, Result, 1)                Call TakeInvItem(Index, GetPlayerInvItemNum(Index, InvNum), 0)                Call PlayerMsg(Index, "You have successfully created " & Trim(Item(Result).Name) & ".", White)              Else                Call PlayerMsg(Index, "You do not have all of the ingredients.", White)                Exit Sub              End If            Else              Call PlayerMsg(Index, "You do not have all of the ingredients.", White)              Exit Sub            End If          Else            Call PlayerMsg(Index, "This is not the tool used in this recipe.", White)            Exit Sub          End If Link to comment Share on other sites More sharing options...
Robin Posted September 21, 2010 Share Posted September 21, 2010 That's the wrong procedure. That's "HandleAttack". You need "HandleUseItem". >_> Link to comment Share on other sites More sharing options...
Dzastin Posted September 21, 2010 Share Posted September 21, 2010 Thanks Robin, I Love You ;DThe script works, thanks:) :renzo: Link to comment Share on other sites More sharing options...
Murdoc Posted September 22, 2010 Share Posted September 22, 2010 This is a nice feature. Great job! Link to comment Share on other sites More sharing options...
Icie Juicy Posted September 22, 2010 Share Posted September 22, 2010 Great job man, thanks for sharing it with us. I'll poke around on it and try to learn a few things about how to make my own. Link to comment Share on other sites More sharing options...
erkro1 Posted November 1, 2010 Share Posted November 1, 2010 Wow, very usefull :cheesy: Link to comment Share on other sites More sharing options...
willscarlet Posted December 1, 2010 Share Posted December 1, 2010 Hello I had the error message "Must have start up form or sub main()". I recieved this error message when following the instructions here, on the second step. I opened up modConstants and it would not allow me to see the code or the objects, so I compiled it to view the code, when I compile. that error comes up and it still will not allow me to see the code, can you please tell me what I am doing wrong?Thank you all very much for your time and help. Link to comment Share on other sites More sharing options...
Helladen Posted December 1, 2010 Share Posted December 1, 2010 The code looks fine. You should made sure the numbers of the item types match and do not repeat. Recs most of the time will match the client and server. So make sure you finish the tutorial before you test it. Link to comment Share on other sites More sharing options...
Guest Posted December 10, 2010 Share Posted December 10, 2010 FraRecipe doesn't show up for me. Added it to the lists, etc, but it doesn't show when the item type is Recipe, any ideas? Link to comment Share on other sites More sharing options...
Helladen Posted December 11, 2010 Share Posted December 11, 2010 You need to make sure the frame is visible, if it is not, double click on the combo for item types. Then put a check if it is recipe then make the frame visible or something of the sort. Link to comment Share on other sites More sharing options...
Guest Posted December 11, 2010 Share Posted December 11, 2010 @Helladen:> You need to make sure the frame is visible, if it is not, double click on the combo for item types. Then put a check if it is recipe then make the frame visible or something of the sort.Yep did that, still doesn't show up. Link to comment Share on other sites More sharing options...
Helladen Posted December 11, 2010 Share Posted December 11, 2010 Make sure it's not attached to another frame. Like try to move it, and if you can't move it off the frame that its below then you need to cut it and paste it and again place it. Link to comment Share on other sites More sharing options...
Guest Posted December 11, 2010 Share Posted December 11, 2010 @Helladen:> Make sure it's not attached to another frame. Like try to move it, and if you can't move it off the frame that its below then you need to cut it and paste it and again place it.Ah yeah that was the problem, thanks Helladan.EDIT: Now I'm getting a subscript out of range on the line: .lblItemDescStats.Caption = "A " & Trim$(Item(Item1).name) & " and " & Trim$(Item(Item2).name) Link to comment Share on other sites More sharing options...
Helladen Posted December 11, 2010 Share Posted December 11, 2010 Add an editor index check at the top of the sub…If EditorIndex > 1 or EditorIndex < MAX_ITEMS Then Exit sub Link to comment Share on other sites More sharing options...
Guest Posted December 11, 2010 Share Posted December 11, 2010 That gets rid of the DescWindow all together O_o. Link to comment Share on other sites More sharing options...
Helladen Posted December 12, 2010 Share Posted December 12, 2010 I don't know then… I'm tired of trying to help you solve a problem that should take 3 seconds to figure out.lbldescstats is something in the frmmaingame that displays description information for items, so you need to check the values it exports, use the debugger...2 things could cause this to happen, 1 your calls are wrong or 2 the editor isn't saving those item values correctly... Link to comment Share on other sites More sharing options...
Guest Posted December 12, 2010 Share Posted December 12, 2010 Meh I just removed it and made a new label on the DescWindow. Thanks for the help anyways. Link to comment Share on other sites More sharing options...
McCull96 Posted December 15, 2010 Share Posted December 15, 2010 nice feature Link to comment Share on other sites More sharing options...
s3th Posted December 28, 2010 Share Posted December 28, 2010 i am using origins xmas and i cant find Handle Use Item….where can i add the code than? Link to comment Share on other sites More sharing options...
Guest Posted December 29, 2010 Share Posted December 29, 2010 Because its Sub HandleUseItem no spaces. Link to comment Share on other sites More sharing options...
Robin Posted December 29, 2010 Share Posted December 29, 2010 The item use code has been moved over to an isolated procedure as well. Link to comment Share on other sites More sharing options...
amadman Posted January 4, 2011 Share Posted January 4, 2011 @s3th:> I am using origins xmas and I cant find Handle Use Item….where can I add the code than?I am in the same boat using Orgins Xmas.I found the HandleUseItem sub but there is no select statement to put the case into.Would this part have to be its own sub now or something?I really have no idea on how to make this work and I would really appreciated it if someone could give me a little push in the right direction. Link to comment Share on other sites More sharing options...
Robin Posted January 4, 2011 Share Posted January 4, 2011 @amadman:> I am in the same boat using Orgins Xmas.> > I found the HandleUseItem sub but there is no select statement to put the case into.> > Would this part have to be its own sub now or something?> > I really have no idea on how to make this work and I would really appreciated it if someone could give me a little push in the right direction.@Robin:> The item use code has been moved over to an isolated procedure as well. Link to comment Share on other sites More sharing options...
amadman Posted January 4, 2011 Share Posted January 4, 2011 Thanks Robin.I read that before and honestly at this point don't exactly know what it means. Where would I look for that at?I looked through the modhandledata and did not see anything other than the HandleUseItem sub. Link to comment Share on other sites More sharing options...
Recommended Posts
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now