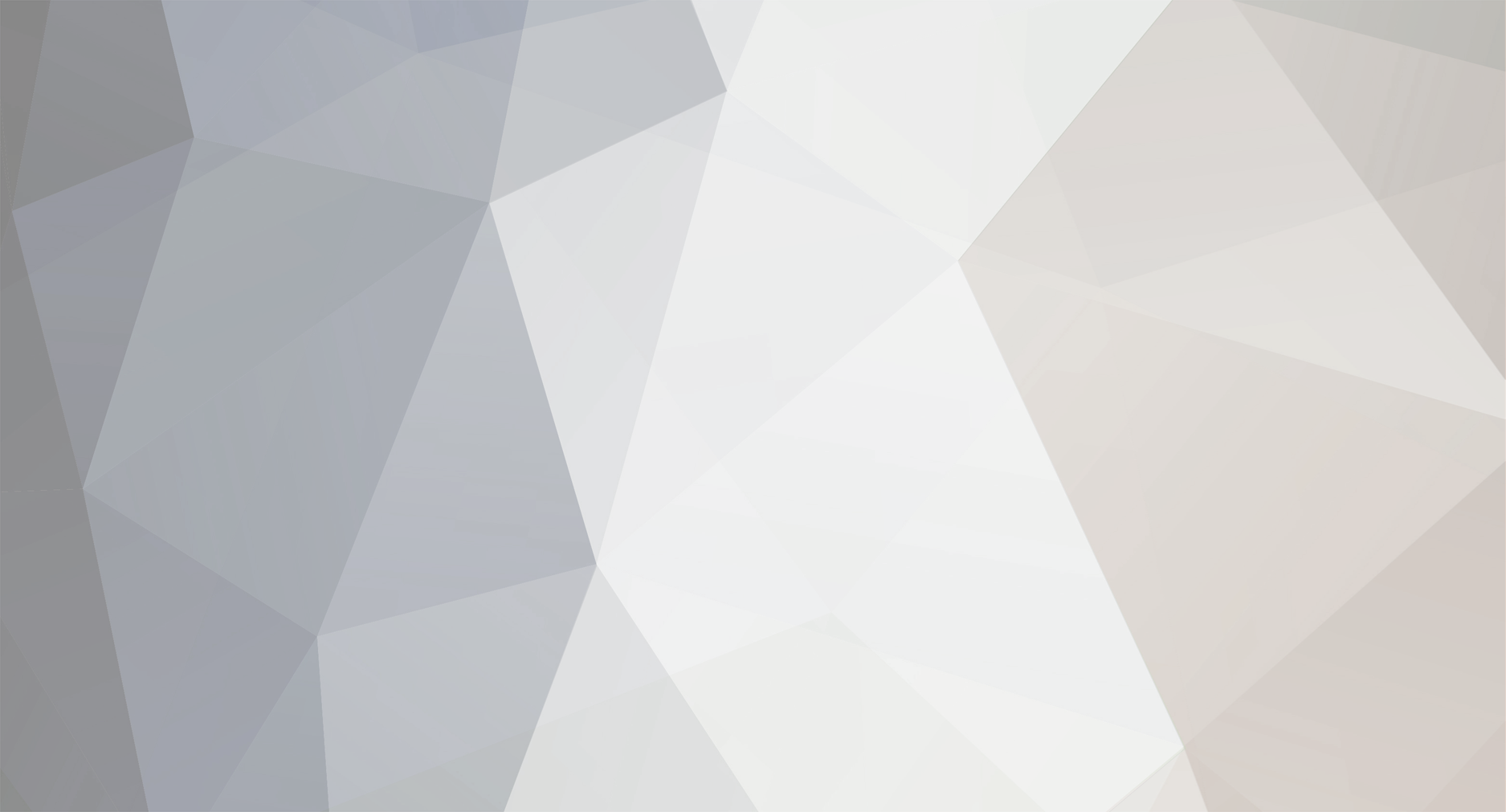
DarkMazer
Members-
Posts
107 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Everything posted by DarkMazer
-
If you really, really want to know how to do it, here's how it works: Do a Select Case statement with the player's direction being the variable, then for each case check to see if there's an NPC at the map coordinates right in front of the player, and if there is an NPC there, damage it, and do whatever else you want. Of course, to check if an NPC is in front of the player, you're probably going to have to use a loop and check each NPC index on the map from 1 to 15, and see if that NPC's X and Y coordinate match the space in front of the player. There are ways to repeat less code, but those involve more mental effort and a good understanding of variables. It's a headache to deal with, and adding something to OnAttack to check an item's number is much easier.
-
You're welcome :cool: There's already a built-in timer feature (see http://www.touchofdeathforums.com/smf/index.php?topic=16378.0). There's also a GetTimer("name of timer") command not covered in there that returns how many milliseconds until the timer executes. This kind of timer executes something after a delayed amount of time (like an attack that takes effect 2 seconds later, or removing the effects of a buff spell after 1 minute). The main reason I can see to use ScriptGetTickCount is to allow someone to do something only once in a certain amount of time (like a cool-down time). Still, many MMORPGs use cool-down timers, and there are many uses for them.
-
Function ScriptGetTickCount() just returns how many ticks (milliseconds) have passed since either the computer or the server started up (can't remember which off the top of my head). Let's see if I can make an example… ``` Sub Boom(index) If Int(GetVar("ticks.ini", GetPlayerName(index), "Ticks")) + 5000 > ScriptGetTickCount() Then Call PlayerMsg(index, "Wait longer", RED) Else Call PlayerMsg(index, "BOOM!", YELLOW) Call TickStore(index) End If End Sub Sub TickStore(index) Call PutVar("ticks.ini", GetPlayerName(index), "Ticks", ScriptGetTickCount()) End Sub ```Now, if you ignore any annoying, reasonless bugs that might be present, that should work. Basically, Sub Boom will say "Boom!" when called, but only if it's been 5 seconds since it was last called. Otherwise, it'll say "Wait longer". Anyways, if that doesn't work, try using ScriptGetTickCount instead of ScriptGetTickCount(). I'm not exactly an expert on proper syntax.
-
I can't believe I just noticed this… > If Clicked_Index = 2 From this point on in your MenuScripts sub, you forgot the Then after your If statements.
-
Public Sub MovePlayer(ByVal index As Long, ByVal Direction As Long, ByVal Movement As Long) Direction is a number between 0 and 3 (0 is up, 1 is down, 2 is left, 3 is right), and I think Movement is either "walk" or "run".
-
It might be auto-truncating the decimal, so 1.5 becomes 1, since SetSpeed only takes in integers. Other than that, from what I've seen, it won't work with numbers that aren't powers of 2. I tried setting run speed to 6 once, and it didn't change.
-
It won't work for any other numbers.
-
That looks like the way it works. Paperdoll will only be rendered on a player if paperdoll is set to 1 in data.ini and the player's paperdoll value is 1. PS. This is the complete list of commands, as of EE 2.7: http://www.touchofdeathforums.com/smf/index.php?topic=28153.0
-
SetSpeed only works with powers of two, and only between 1 and 32. A complete list of numbers that work is 1, 2, 4, 8, 16, and 32.
-
There was a thread about this exactly in the script request forum. [http://www.touchofdeathforums.com/smf/index.php?topic=34707.0](http://www.touchofdeathforums.com/smf/index.php?topic=34707.0)
-
Are you using pictures for your menus? If not, then you're putting all of those ifs in the wrong place, and they need to be moved to case 3.
-
GetPlayerWeaponSlot returns the slot in your inventory that your equipped weapon is in. Try this: If GetPlayerInvItemNum(index, GetPlayerWeaponSlot(index)) = 2 Then … End If That'll give you the item number of the player's weapon.
-
A quick search turned this up: Left: 37 Up: 38 Right: 39 Down: 40 Couldn't you just use LockPlayer instead, though?
-
The problem is this: > "Type "Yes" or "No" to answer" It doesn't think those quotes in the middle are quotes, it thinks they're the beginning and end of strings… The proper way to do it is: > "Type ""Yes"" or ""No"" to answer" At least, I think that's the only problem… Also, unless I'm mistaken, text boxes in EE 2.7 won't take in string input anymore, because it could be exploited to run scripts...
-
@Dastyruck: > Sub HOTSCRIPT1(index) > > Does not work in 2.7, is there a replacement for it? Sub HotScript(index, 1) should do the same thing. Just put everything you would have put in HOTSCRIPT1 into case 1 in Sub HotScript.
-
There were a few things that got messed up when you copied the script over. > Call PutVar("mailsystem\" & reciever & ".ini", "mailbox", "box" & n, > > GetPlayerName(index) & ": " & field_message & "") That should all be on one line. Same with: > 'Call playermsg(index, "Clicked field number " & clicked_index & " on the menu named " & menu_title & ". The field text was > > " & field_message, 14) Also, after this: > '****************************************************************** > ' Executes when a hotkey is pressed client-side. > Sub HotScript(Index, KeyID) > '****************************************************************** You need to add this: > Select Case KeyID > Case 1 And here: > Call CustomMenuTextBox(index, 1, 180, 65, 235, "Enter a name!") > Call CustomMenuTextBox(index, 2, 180, 65, 260, "Enter a message!") > End Sub You need to delete the line that says End Sub. Hopefully that should get you closer to having a working script.
-
@KDawg08: > so with this script it'll be Case 1 as it is now, BUT the extra case #'s on there are for each seperate NPC? and they walk around so… would that be a problem also? They're nested select case statements. The ones on the inside don't affect the ones on the outside at all. The script executes the moment you hit them with an attack, so the only reasons this script shouldn't work are if you're attacking them from a distance, or if your server has really bad lag.
-
It already has hotkeys for PageUp, PageDown, End, and Home. Sub HotScript is what it's called. It's just a tad different in that it contains all 4 cases in one sub. Still, translating it should be easy, since Sub HotKey1 is now case 1 in Sub HotScript, etc. The rest should already be there in unaltered form.
-
You got me curious about this. So far, I can tell that GetNpcName(NPCNum) is used to get the NPC's name, and GetMapNpcNum(mapnum, NPCindex) is used to get the NPC's number for the GetNpcName command, but I can't figure out how to get the NPC's index on that map, since it's not included in Sub ScriptedNpc, and attacking a scripted NPC won't make it your target, apparently… If anyone has any ideas on the last bit, feel free to step in here.
-
Rand(10, 1) should be Rand(1, 10). It works the first way in TE, but it was changed to the second way in EE. Also, GetVar("Words.ini", "Words", ""& Words &"") should be GetVar("Words.ini", "Words", "Words" & Words)
-
Double attack (not double dmg) Help-p10x!
DarkMazer replied to mrmiguu's topic in Programming Questions
@MrMiguuâ„¢: > defined in the spell case? and put the attack time in the settimer thing? (edit the & Attacks & to & 2 &?) Yep, they need to be defined in the spell case, if they're not already. The script would probably end up looking like this: ``` if GetPlayerTarget(index) > 0 And GetPlayerTarget(index) index then Call SetTimer("MakeAttackP " & index & "," & damage & "," & Target & ", 2", 100) else Call SetTimer("MakeAttackN " & index & "," & damage & "," & Target & ", 2", 100) end if ```I made the time 100 milliseconds because that's what you said you wanted earlier. For the attack thing, I just made Attacks 2 and then took out the &, but that should make it work. -
Double attack (not double dmg) Help-p10x!
DarkMazer replied to mrmiguu's topic in Programming Questions
In this part: ``` if GetPlayerTarget(index) > 0 And GetPlayerTarget(index) index then Call SetTimer("MakeAttackP " & index & "," & damage & "," & Target & "," & Attacks, 200) else Call SetTimer("MakeAttackN " & index & "," & damage & "," & Target & "," & Attacks, 200) end if ```You have to fill in Attacks with how many attacks you want to make (2, probably). You also have to make sure Index, Target, and Damage are defined properly. -
@oturaz: > in TE 2.7 ….. :sad: I hope you mean EE 2.7… That's because of a bug that makes any spell that's not an area spell not work properly. It's one of the only bugs I find constantly in EE 2.7, but it's still a pain. Also, one post is enough. You don't have to post the exact same thing 4 times. That's actually considered spamming.
-
I'm having a lil bit of trouble
DarkMazer replied to SpaZ The Impaler's topic in Programming Questions
In EE 2.7 (I assume that's what you're using now), Sub OnAttack only executes when you hit an enemy from right next to them. Try moving all of the ranged weapons into Sub OnArrowHit. -
Using DamageNPC also makes the attacking character do the attack animation, but since it is usually used with a spell, and the attack animation is already being shown, that doesn't make much of a difference.