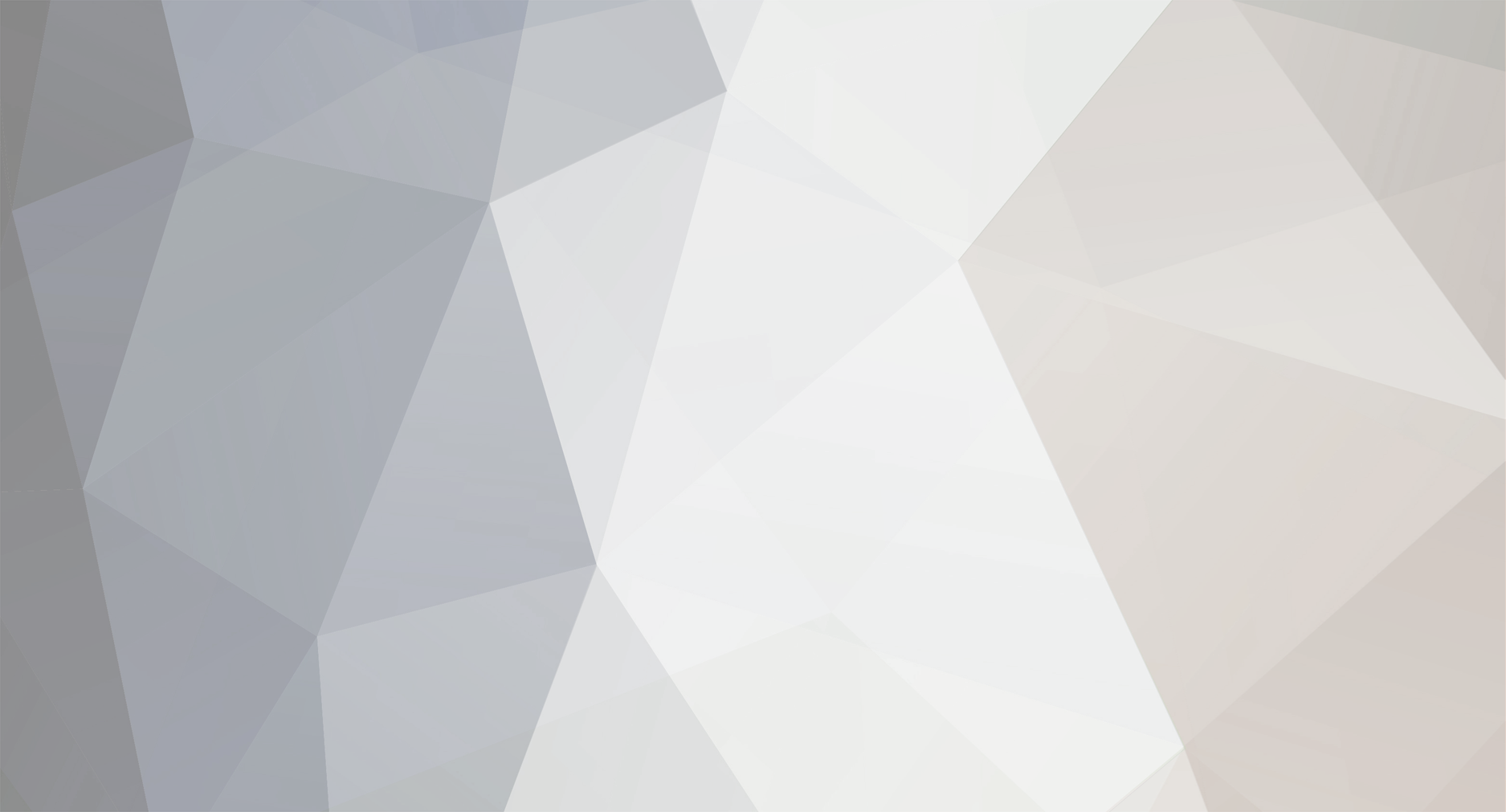
Homicidal Monkey
Members-
Posts
3997 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Everything posted by Homicidal Monkey
-
got bored today, so I optimized a few functions in EO2.0 server/client server change log: -greedy buffer allocation -on-demand player timers client change log: -sorts character/npc/resources by y before rendering client & server are compliant with EO2.0 **Current build** [Download + Source](http://www.freemmorpgmaker.com/files/imagehost/pics/74b97929f8b65d43474d7220f6644939.zip) **Old builds** [b12.04.12: has possible y-bounds error](http://www.freemmorpgmaker.com/files/imagehost/pics/f0a43eee5de0ed4db0f4a00c5c6e162f.zip) how it works: >! original method implemented allocated room when needed and only allocated the amount needed. Assuming vb6 memory allocations are based on C memory allocations, growing an array actually results in: >! * creating a new array * filling the array with the old data * deleting the original array >! greedy method doubles the size of the buffer when more room is needed and then shrinks the buffer. Again, assuming vb6 memory allocations are based on C memory allocations, this results in: >! * creating a new array * filling the array with old data * deleting the original array >! so what's the difference? The passive-growth method has to reallocate the array every time a new element is added, while the greedy-growth method only has to when its out of room. >! PS: assuming shrinking array uses similar algorithms to realloc in c, shrinking the array should run in O(1) time. >! vanilla EO2.0's serverloop iterates through all the players at each loop interval when the timer is required for refresh. The only problem here really is that the significant digits is seconds, not 25ms. So instead of iterating each player's timers every 25ms, it iterates 1 player timer every loop. This results in a cost function: 25*O(1) where as original had O(n). >! significance: input n>25 and it runs better. (where n is number of players). This method was implemented in a few other areas involving timers. >! to properly display characters, EO2.0 iterates across a maps y-axis and then iterates across each character, npc, resource. >! why this is bad: assume tiles-down is 11 and 75 players (predefined max). This results in a total of 75*75*75*75*…*75 = 75^11 operations just to check if it needs to draw a player. (its 30^11 for npc, no idea what max is for resoures) >! how it works: counting sort. for each y value, it counts each character, npc, resource at that location and saves the index. This results in (assuming 75 characters, 30npc, 10 resources, 11 tiles-down): reset arrays: 3*11 counting: 75 + 30 + 10 drawing: 75 + 30 + 10 >! I haven't been able to test performance gain for this, but it should be significant if you have over 2 players on a map. 1 player on a map renders at approx same speed as before. Assuming internet isn't limiting factor, you could (in theory) have a map with 1 character per square, without lagging. >! note: bandwidth consumption in Eclipse is still a n^2 function. Also, this optimization is more in-place for potential reports. vb6 2D arrays are horribly optimized I'll do some more fixes later, maybe. From what I remember, AI is horrible.
-
>! ``` .data msg: .asciiz "Enter an integer: " endl: .asciiz "\n" .text >! main: jal readInput add $a0, $v0, $zero #move result to parameter 0 jal printAsterics >! li $v0, 10 #exit syscall >! #reads input, returns result in $v0 readInput: li $v0, 4 la $a0, msg syscall li $v0, 5 syscall jr $ra >! #prints row of asterics #parameters: # a0: rows/cols to print #returns: # v0: 0 if ends properly printAsterics: add $t9, $zero, $zero #rows >! #inner loop used by printAsterics #prints each row rowLoop: add $t0, $zero, $zero #iterator sub $t1, $a0, $t9 blez $t1, endRow >! #inner loop of rowLoop #prints each asteric in the row astericLoop: #check if t0 > a0 sub $t1, $a0, $t0 blez $t1, endAsterics #jump to end of loop >! #push a0 onto stack (because we need to call a print) addi $sp, $sp, -4 sw $a0, 0($sp) >! #print the asteric li $v0, 11 li $a0, '*' syscall >! #restore a0 lw $a0, 0($sp) addi $sp, $sp, 4 #increment the iterator and loop addi $t0, $t0, 1 j astericLoop >! #end of asteric printing loop endAsterics: #push a0 onto stack (need to print endl) addi $sp, $sp, -4 sw $a0, 0($sp) >! #print endl li $v0, 4 la $a0, endl syscall >! #restore a0 lw $a0, 0($sp) addi $sp, $sp, 4 addi $t9, $t9, 1 #loop the row j rowLoop >! #reached the end of the function, so return endRow: li $v0, 0 #clear v0 jr $ra ``` >! ``` .data msg: .asciiz "Enter an integer: " msg2: .asciiz "Enter an character: " endl: .asciiz "\n" .text >! main: jal readInput add $a0, $v0, $zero #move result v0 to parameter 0 add $a1, $v1, $zero #move result v1 to parameter 1 jal printAsterics >! li $v0, 10 #exit syscall >! #reads input, #returns: # v0: num asterics # v1: char instead of asteric readInput: li $v0, 4 la $a0, msg syscall li $v0, 5 syscall >! add $t0, $v0, $zero #copy result to t0 li $v0, 4 la $a0, msg2 syscall li $v0, 12 syscall >! add $v1, $v0, $zero #v1 = char to print add $v0, $t0, $zero #restore v0=num asterics jr $ra >! #prints row of asterics #parameters: # a0: rows/cols to print #returns: # v0: 0 if ends properly printAsterics: add $t9, $zero, $zero #rows >! #inner loop used by printAsterics #prints each row rowLoop: add $t0, $zero, $zero #iterator sub $t1, $a0, $t9 blez $t1, endRow >! #inner loop of rowLoop #prints each asteric in the row astericLoop: #check if t0 > a0 sub $t1, $a0, $t0 blez $t1, endAsterics #jump to end of loop >! #push a0 onto stack (because we need to call a print) addi $sp, $sp, -4 sw $a0, 0($sp) >! #print the asteric li $v0, 11 add $a0, $a1, $zero syscall >! #restore a0 lw $a0, 0($sp) addi $sp, $sp, 4 #increment the iterator and loop addi $t0, $t0, 1 j astericLoop >! #end of asteric printing loop endAsterics: #push a0 onto stack (need to print endl) addi $sp, $sp, -4 sw $a0, 0($sp) >! #print endl li $v0, 4 la $a0, endl syscall >! #restore a0 lw $a0, 0($sp) addi $sp, $sp, 4 addi $t9, $t9, 1 #loop the row j rowLoop >! #reached the end of the function, so return endRow: li $v0, 0 #clear v0 jr $ra ``` >tested in spim I'll post solutions in other languages later
-
have you opened it yet to see the size of connectors to the capacitors? btw, this thread still reeks heavily of ishygddt
-
its probably going to be impossible to do. A monitor is going to have multiple capacitors, also capacitors have different thresholds and discharge rates. It is totally possible for the monitor to have custom-built capacitors or capacitors of varying rates/thresholds. Also, if its the powersupply, I'd suggest not touching that. source: I've shocked myself on a 300v capacitor once. It hurts. Also I've taken plenty of E/M classes
-
I can't believe I actually missed the April Fools day prank. Eclipse always had the most balls-deep april fools day pranks
-
lololol your like 2 years late to this.
-
wtfisthis, idonteven
-
@Adulese: > Oh hey HM. I haven't seen you in a while mate. You need to jump on MSN every now and then. > > - Adulese you're never on when I'm on
-
Games everyone should play at least once in their lifetime
Homicidal Monkey replied to Gwen's topic in Video Games
Earthbound >!  Secret of Evermore Chrono Trigger Battle Toads -
@Zonova: > Umm, we released Eclipse in Java? lol
-
@Draken: > there was a great battle between gnomes and marshmallows then all of the sudden a penguin army came out of no where and the gnomes and marshmallows allied against the new foe and became victorious. > > other then that nothing much so 2007 happened again
-
INTJ, lowest score was 70% (J). mode: ~90 amidoingitright? bonus interesting statistic: majority of 4chan users are INTJ, followed by INTP.
-
@Kamii: > Robin dropped developing, van shaik (i hope i wrote it correctly…) thought about a c version of eclipse. > Well some freaks copied CS:DE engine and say it's theirs.. > Nothing else which is worth a word i think. lol heard about all three via Anthony. @Robin: > lolhi. *waves*
-
gone for like half a year because internets and MIPS. what I miss?
-
@Zonova: > You weren't joking about the heavy graphics thing wut
-
>playing apb >mission: secure car >teammate securing car >be shit driver >accidentally ram car with my car >both cars flipped >he mad
-
done with tutorial. username: fabs this game is just gta for white ppl.
-
@Robin: > You're 12\. Run Windows.
-
Are you sure the song wasn't death metal? 
-
works on my firefox. -appears to not have internets -free returns 16mb of ram -running linux 2.6.20
-
The world is going to end May 21? Yes or No
Homicidal Monkey replied to dpinoy13's topic in Off Topic
I'm not accepting any form of the world ending besides zombie apocalypse. -
I did this once. I think you have to do it on the client side since attacks and stuff are calculated server side. It would end up being a search for nearest npc in specific direction script.
-
@Robin: > trollface.svg I prefer .html