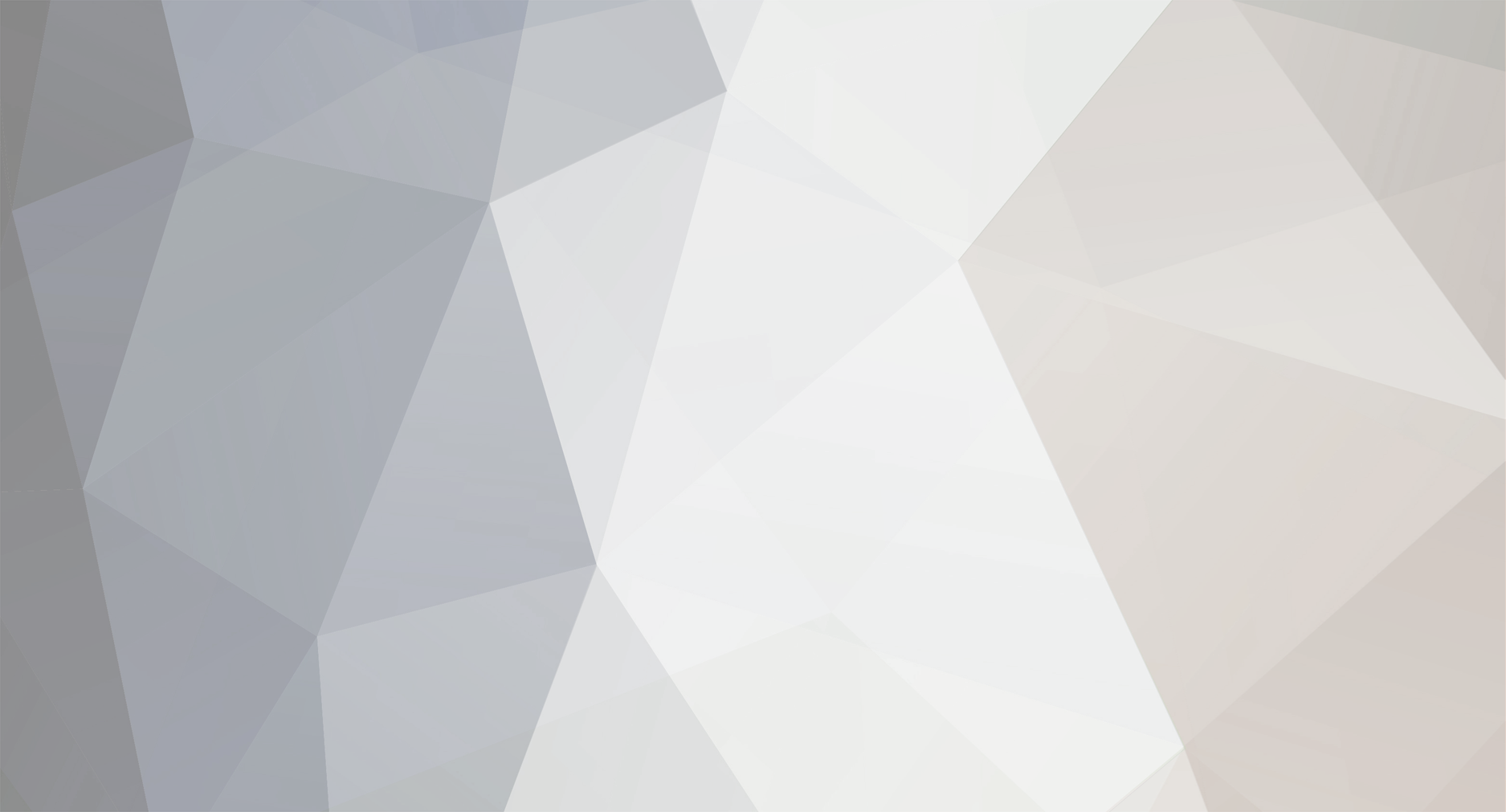
Growlith1223
Members-
Posts
2042 -
Joined
-
Last visited
Content Type
Profiles
Forums
Calendar
Everything posted by Growlith1223
-
In normal versions of Eclipse(for those who have no idea what im talking about, the engine uses GetTickCount directly instead of checking to see if the value is = to or < than 0) the Tick value can go lower than 0 and cause the engine to crash from Longs not being able to use negative values. To fix this it's rather simple, and I'll show you how! :D OK, so first thing you should do is decide to use timeGetTime instead because it will last longer, and its more accurate. the next thing you should do is go into where you have your server/client loop and copy/paste this in: ``` Public Function GetTick() As Long Const MAX_INT As Long = 2147483647 If GetTickCount < 0 Then GetTick = MAX_INT + GetTickCount Else GetTick = GetTickCount End If End Function ``` after that, go into said loop and find where it says Tick = GetTickCount. and change that GetTickCount to just GetTick! you're for the most part done, you will have to find all the areas that are calling GetTickCount and change it to that GetTick function! this will ensure the value is ALWAYS a positive value to ensure that it won't error after a long host(keeping the server up for days would eventually crash it due to said negative value). Anywho, lemme know if you get confused by this tutorial and i'll help you in any way I can!
-
ah, this shouldn't change anything, I haven't tested it though but it looks good!
-
@'Jaxx': > Dang xD I thought I was being so clever with this 'fix'. > > Hmmm, well we'll see if I use it in EOO. As for everyone else, it's here for use haha. owait nvm, ignore what I am saying, I thought this was modifying the movement speed, looking into this further, it looks good for what its doing but I actually don't see any difference using timeGetTime vs this method.
-
im not saying that it's not good, just if the system lags, that timer will jump if I remember correctly, causing the same issue as before, it's a very small chance the system WOULD lock up from anything but it can happen lol
-
@'Jaxx': > @'Growlith1223': > > > Time based movement within the eclipse engine was more or less an expirement and turned out to be a failure. regardless, good tutorial, I don't see anything wrong with what you have here! > > What was wrong with Time based movement? Does it not solve the problem of lag-moving? It would cause the player to jump around beyond belief, even with a good computer mind you, the client would desync a lot and cause other players to be at like x 10, to x 33 or something, lol
-
Time based movement within the eclipse engine was more or less an expirement and turned out to be a failure. regardless, good tutorial, I don't see anything wrong with what you have here!
-
@'DarkDino': > Make this little changes to optimize :3: > > ``` > 'write name & level & hp > For i = 1 To Npc_HighIndex > if mapNpc(i).num > 0 And Not myTarget > Npc_HighIndex Then > If MapNpc(myTarget).num = mapNpc(i).num Then > RenderText Font_Default, NPC(MapNpc(myTarget).num).Name, x + 10, y + 4, Yellow > RenderText Font_Default, MapNpc(myTarget).Vital(HP) & "/" & NPC(MapNpc(myTarget).num).HP, x + 55, y + 15, Red > RenderText Font_Default, NPC(MapNpc(myTarget).num).Level, x + 150, y + 4, Yellow > Exit For ' Leave the sub when this drawed > End If > End if > Next > > ``` You don't need the for loop lol, it's already rendering to myTarget and it's already checking before the sub is even being called lol, just a waste of cpu power
-
@'Morikem': > @'Growlith1223': > > > Your Runtime error revolves around an index that's higher or lower than the current maximum. if you have VB6, breakpoint on the login sequence, and see what the GuildNum is, and see if it's higher than the current maximum(would be like Guild(1 To MAX_GUILDS) As GuildRec). I can fix this for you but I don't do free work, sorry > > Right so: > > Find the login sequence (ModClientTCP?) > Check that the guild number isn't higher than the maximum of guilds. (ModGuild) > > Seems straightforward enough, I'll take a look at it after dinner. > I'm starting to get a feel for Eclipse in VB6. If you don't know how to breakpoint, go to a line of code, and move your mouse to the very left, the cursor should flip or just not have the text cursor, after that just click, the application should pause at the exact line you set the breakpoint. just see what GuildNum is equal to, and see what MAX_GUILDS is also equal to, and go from there :P
-
@'Kitam': > What name is news graphics? ( in Gui folders) :P RenderTexture Tex_GUI(21), look for 21.png in the gui foder
-
lol it happens, I have had so many of these moments xD and no problem! im always here if you or anyone else needs help :P
-
IMO, the chatbox should stay on the main forum page, but I don't mind the chatbox everywhere
-
Your Runtime error revolves around an index that's higher or lower than the current maximum. if you have VB6, breakpoint on the login sequence, and see what the GuildNum is, and see if it's higher than the current maximum(would be like Guild(1 To MAX_GUILDS) As GuildRec). I can fix this for you but I don't do free work, sorry
-
You might want to look into removing the for loop within your code, it's unnecessary and just adds into the cpu usage, especially since said for loop is literally checking to see if npcnum is more than 0 and is constantly called 10-20 times every "second"
-
> ~~Does it matter if it get's cleared every time? Because all I'm checking for is if it's less than GetTickCount. Even if cleared, the new number would still be less than GetTickCount. And then it will jump up to GetTickCount + 30ms after.~~ > > > > Wait a minute! Just realized how the render is called. >.< I'm an idiot. I'm going to just stop thinking for the night xD I'll post an updated source tomorrow morning. lol, it happens :P
-
> Ohhh Okay. Sorry I didn't see your point sooner, I must be pretty tired. Yeah, you're right. > > ``` > Public Sub DrawTargetDetails() > Dim i As Long, x As Long, y As Long > Dim Width As Long, Height As Long > Dim UpdateTimer as Long > > ' render the window > Width = 256 > Height = 64 > ' render the window on screen > x = 300 > y = 64 > > ' render the details > RenderTexture Tex_GUI(24), x, y, 0, 0, Width, Height, Width, Height > > If UpdateTimer < GetTickCount then > 'write name & level & hp *update* > RenderText Font_Default, NPC(MapNpc(myTarget).num).Name, x + 10, y + 4, Yellow > RenderText Font_Default, MapNpc(myTarget).Vital(HP) & "/" & NPC(MapNpc(myTarget).num).HP, x + 55, y + 15, Red > RenderText Font_Default, NPC(MapNpc(myTarget).num).Level, x + 150, y + 4, Yellow > UpdateTimer = GetTickCount + 30 > End if > > End Sub > > ``` > There, that should fix the problem while also keeping the Target Vitals updated. I didn't test this and I'm pretty tired, so I may have missed something. xD that still won't work lol, UpdateTimer would get cleared the moment the sub goes out, technically speaking you'd want the timer as a public variable(in like modglobals or modGameLogic if you want to get organized) and store it there(although i would just put the tick check around the call check instead but this works too), doing what you have now would always say if UpdateTimer(which = 0 everytime) < Tick Then. it would act like that statement isn't even there lol sorry, just nit picky with code sometimes
-
> I'd say it's still checking to make sure it's an NPC at all. There doesn't seem to be any code for checking other players. It need to be rendered constantly because the health may change, yes? > > > > Unless I'm just super tired and missing something. That's not what i mean when the sub is actually called it's making a check to see if it's an NPC to begin with, after that, it goes into a for loop through NPC_HighIndex, meaning every npc within the map, this means, even if it's not the npc we're targeting, it's still calling DrawText, now mind you, 1 or 2 npcs you won't notice anything, throw nearly 30 to 40 in, and you'll start to see a drop in some performance and a bit more cpu usage since it's calling 3 DrawTexts for EVERY npc, not just the npc we're targeting. the constant rendering is done inside Render_Graphics. all im saying is, that for loop is going to kill the cpu with unnecessary calls EDIT: and even if it does need to be updated constantly, it doesn't need to be updated to the absolute ms, it can be thrown into a small timer(about 15-60ms timer) and it will still be fast enough to read perfectly fine.
-
@[member="SkywardRiver"] You must be thinking of the check before the actual call is made For loop is constantly drawing the same thing, i say this because it's not actually checking to see if it's the correct npc, it's just checking to see the ncpnum is higher than 0, and if so, render, essentially this is rendering nearly 10-20(depending on how many npcs there are) times everytime it's called lol ``` 'write name & level & hp For i = 1 To Npc_HighIndex ' Go through every possible npc within the map If MapNpc(i).num > 0 Then ' Check if MapNPC Num is more than 0, if so, proceed RenderText Font_Default, NPC(MapNpc(myTarget).num).Name, x + 10, y + 4, Yellow RenderText Font_Default, MapNpc(myTarget).Vital(HP) & "/" & NPC(MapNpc(myTarget).num).HP, x + 55, y + 15, Red RenderText Font_Default, NPC(MapNpc(myTarget).num).Level, x + 150, y + 4, Yellow End If Next ``` it just looks highly innefficient to me
-
just a question because i can't figure it out for the life of me, but why are you doing a for loop? it doesn't seem to be of any use inside of the code here. this is using myTarget, not i lol, from what i see, I is to check for nothing? sorry, i might be just not seeing the use for it xD
-
> @[member="Growlith1223"] do you also work in VB6 also welcome back! Thank you and yes, i also work in VB6! I also know a bit of C# but not enough to actually start accepting offers for said language lol
-
I have returned and am now looking for work again! I can safely say now i work in VB.net more.
-
KabukiSDK(C# And VB.Net) v1.0 (Open Source)
Growlith1223 replied to ArchaicOokami's topic in Programming
> By far superior to anything you'll ever write. To each their own -
[http://www.eclipseorigins.com/community/index.php?/topic/136067-compile-issue-with-eclipse-worlds/](http://www.eclipseorigins.com/community/index.php?/topic/136067-compile-issue-with-eclipse-worlds/) [http://www.eclipseorigins.com/community/index.php?/topic/136171-vb6-server-start-error/](http://www.eclipseorigins.com/community/index.php?/topic/136171-vb6-server-start-error/) [http://www.eclipseorigins.com/community/index.php?/topic/135504-error-with-a-new-engine/](http://www.eclipseorigins.com/community/index.php?/topic/135504-error-with-a-new-engine/) [http://www.eclipseorigins.com/community/index.php?/topic/135412-i-have-somes-problems-with-my-vb6/](http://www.eclipseorigins.com/community/index.php?/topic/135412-i-have-somes-problems-with-my-vb6/) You don't search much do you?
-
Here's mine: >! CPU: Intel Core i5 4690k Quad Core processor overclocked to 4.7GHz($212.99) >! Motherboard: Asrock Z97 Fatal1ty Killer($129.99) >! RAM: 8GB(2 x 4GB) Crucial Ballistixs 1300Mhz($79.99) >! HDD: Seagate 1TB 7200RPM($54.88) >! SSD: Some random 250GB i found in my old laptop >! GPU: Zotact AMP! Edition GTX 760($214.99) >! Case: Thermaltake V3 AMD Edition($59.99) >! PSU: Rosewill Green Series 630W($59.98) >! OS: Windows 7 Ultimate 64-bit($197.96) >! Monitor: Samsung S19B220NW 19"($159.99) >! Total: $1197.74
-
Disappointment of new ownership decision and Concerns.
Growlith1223 replied to kingfrost's topic in Off Topic
In all fairness it's your fault for putting such high hopes on an official new engine. Engine's take a lot of time to develop, no matter how small they are. I believe it was cancelled due to the new owner not getting the source from Amish, the previous Emperor. While yes, they did bring a lot of hype into the atmosphere when it was actually being worked on, however that drastically fell when there was literally no development notices whatsoever. Regarding your "hire a new team" comment, take a look around in the talent center and see which programmer works for you, there's many capable of doing what you are asking, for the right price ofc. some people will ask for lower, while others, higher. - Growlith1223