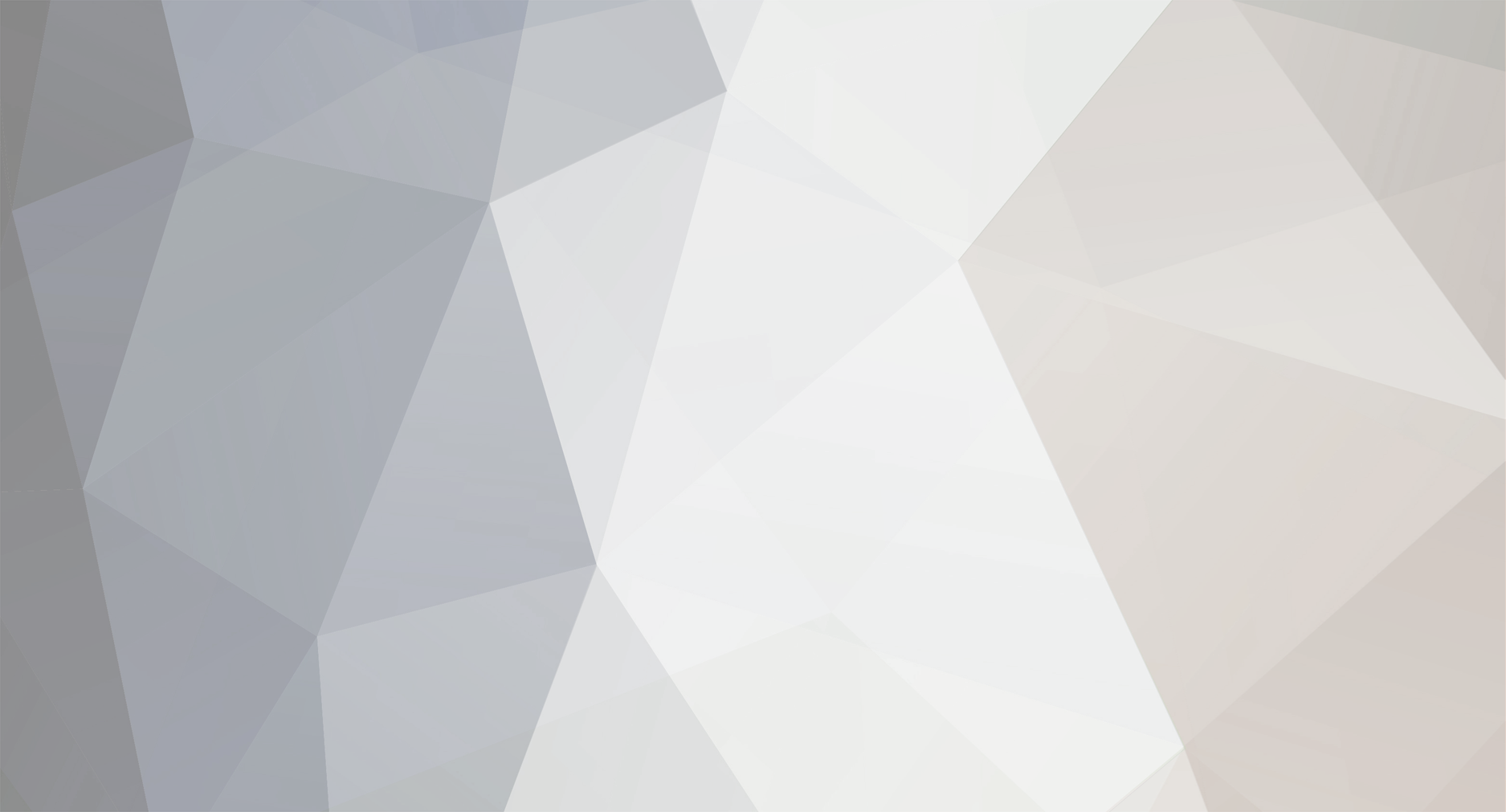
zdearborn
Members-
Posts
84 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Everything posted by zdearborn
-
hey its no problem, I appreciate your help! but, in saymsg: ``` Case NPC_CHAT_PLAYERMSG ' player to npc Buffer.WriteLong SSayMsg Buffer.WriteString GetPlayerName(Index) Buffer.WriteLong GetPlayerAccess(Index) Buffer.WriteLong GetPlayerPK(Index) Buffer.WriteString message Buffer.WriteString "[NPC] " Buffer.WriteLong saycolour Buffer.WriteLong 3 ```(player's msg) is sent before the npc chat subs are called: ``` ' npc chat If Player(Index).inNpcConvo = True Then Call processNpcConversation(Index, npcNum, GetPlayerMap(Index), message, False) ElseIf Player(Index).inNpcConvo = False Then Call instantiateNpcConversation(Index, npcNum, GetPlayerMap(Index), message) End If ```
-
Hmpf, no it does not it will just automatically pass the player msg and then run the checks… so I should just send the player msg back in the processNpcChat sub then?
-
Hm a bit confused about the question, a little thick I guess :P, but I will try to answer, sorry if its way off ``` Case 3 ' npc chat ' make sure player has valid npc targeted If npcNum > 0 And Npc(npcNum).Behaviour = NPC_BEHAVIOUR_SHOPKEEPER Then Call SayMsg_NPCChat(Index, Msg, QBColor(White), 0, npcNum) End If ``` is the HandleSayMsg check…
-
Hello! Today I've begun (trying) to write a NPC chat system which works based off keywords. The server is reacting to the keywords correctly, but there's one problem, the NPC text shows up before the player's does, which creates a problem: the npc is not a mind reader and cannot respond before the actual player msg! :P Here is the code for my NPC processing the keywords: ``` Public Sub processNpcConversation(ByVal Index As Long, ByVal npcNum As Long, ByVal mapNum As Long, ByVal playerMsg As String, ByVal justGreeted As Boolean) Dim distanceX As Long, distanceY As Long, allowedRange As Long, validConvo As Boolean Dim playerName As String ' the range at which the player can talk to the npc allowedRange = 4 distanceX = GetPlayerX(Index) - MapNpc(mapNum).Npc(npcNum).x distanceY = GetPlayerY(Index) - MapNpc(mapNum).Npc(npcNum).y validConvo = True ' checks ;p If Not IsPlaying(Index) Then validConvo = False If Not GetPlayerMap(Index) = mapNum Then validConvo = False If distanceX > allowedRange Or distanceX < -allowedRange Then validConvo = False If distanceY > allowedRange Or distanceY < -allowedRange Then validConvo = False If validConvo = False Then Player(Index).inNpcConvo = False Exit Sub End If ' convenience playerName = Trim$(Player(Index).Name) playerMsg = Trim$(LCase$(playerMsg)) ' npc vars Dim npcGreeting As String, npcBye As String ' messy below... hardcoded npc interaction!! Select Case npcNum Case 2 ' test npc npcGreeting = "Hello, " & playerName & ", welcome to The Barracks!" npcBye = "Bye, then.." If justGreeted = True Then Call SayMsg_NPCChat(Index, npcGreeting, QBColor(White), 1, npcNum) End If Select Case playerMsg Case "barracks" Call SayMsg_NPCChat(Index, "The barracks is where beginners like yourself train before going out to fight for our beloved King.", QBColor(White), 1, npcNum) Case "bye" Call SayMsg_NPCChat(Index, npcBye, QBColor(White), 1, npcNum) Player(Index).inNpcConvo = False Exit Sub End Select End Select End Sub ``` and heres sub saymsg_npcchat ``` Sub SayMsg_NPCChat(ByVal Index As Long, ByVal message As String, ByVal saycolour As Long, ByVal msgType As Long, ByVal npcNum As Long) Dim Buffer As clsBuffer Set Buffer = New clsBuffer Select Case msgType Case NPC_CHAT_PLAYERMSG ' player to npc Buffer.WriteLong SSayMsg Buffer.WriteString GetPlayerName(Index) Buffer.WriteLong GetPlayerAccess(Index) Buffer.WriteLong GetPlayerPK(Index) Buffer.WriteString message Buffer.WriteString "[NPC] " Buffer.WriteLong saycolour Buffer.WriteLong 3 ' npc chat If Player(Index).inNpcConvo = True Then Call processNpcConversation(Index, npcNum, GetPlayerMap(Index), message, False) ElseIf Player(Index).inNpcConvo = False Then Call instantiateNpcConversation(Index, npcNum, GetPlayerMap(Index), message) End If Case NPC_CHAT_NPCMSG ' npc to player Dim npcName As String npcName = Trim$(Npc(npcNum).Name) Buffer.WriteLong SSayMsg Buffer.WriteString npcName Buffer.WriteLong 5 Buffer.WriteLong 0 Buffer.WriteString message Buffer.WriteString "[NPC] " Buffer.WriteLong saycolour Buffer.WriteLong 3 Case Else Set Buffer = Nothing Exit Sub End Select SendDataTo Index, Buffer.ToArray() Set Buffer = Nothing End Sub ```(the added long that at the end is sent for designating which chat channel it goes in, if anyone is confused by that) Now I'm sure I have overlooked something, but I have tried rearranging some code, nothing seems to fix this. To reiterate, the ultimate goal: have the npc text appear AFTER the player's. The client side I'm sure is ok, because it's just a modified version of the original handlesaymsg, but if you need any more code, please just ask and I will post it asap. Here's a screen:  as you can see all responses are displayed before the player msg is the answer is probably so simple I'll feel stupid.. wait, I do feel stupid Thanks for any help!
-
How to write a bot that press everytime f1 in a another window?
zdearborn replied to horsehead's topic in Discussion
I wroote this keypress simulator a while ago (might be better methods out there, but it works :P): it's in c# ``` using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Threading; namespace Simulator { class Program { const UInt32 WM_KEYDOWN = 0x0100; const int VK_F1 = 0x70; [DllImport("user32.dll")] static extern bool PostMessage(IntPtr hWnd, UInt32 Msg, int wParam, int lParam); [STAThread] static void Main(string[] args) { Console.Title = "Keypress Simulator"; Console.WriteLine("Enter the name of the program you wish to send simulated keypresses to (case sensitive): "); string proName = Console.ReadLine(); Console.WriteLine("start keypress simulator? (y/n)"); string input = Console.ReadLine(); int i = 2; if (input == "y") { while (true) { Process[] processes = Process.GetProcessesByName(proName); foreach (Process proc in processes) { PostMessage(proc.MainWindowHandle, WM_KEYDOWN, VK_F1, 0); } // randomize send time int randomA = 10000; // milliseconds int randomB = 30000; // milliseconds Random rand = new Random(); int random; random = rand.Next(randomA, randomB); Thread.Sleep(random); Console.WriteLine("executed at {0} ({1})", random.ToString(), i.ToString()); i++; } } } } } ``` -
I think its very good! As for the comment on the "floating cliffs" I think a good solution is use the top part of your grass border and mask them over the bottom of your cliffs so you can actually see the grass over the cliff, giving that grounded feeling edit: I don't feel like I did a very good job explaining, so I edited your image to show you what I mean:
-
I don't define it as a parameter in Call DrawInfoText("text")?
-
Hello, I'm trying to make a system where everytime I want to notify a player of something, I want it to blit across the bottom of their game screen for a short period of time, I'm testing it out, but this code will not draw any text: Here is the method call in CheckAttack (done just for testing purposes) ``` If ControlDown Then Call DrawInfoText("text") .... ``` and heres DrawInfoText ``` Public Sub DrawInfoText(ByVal text As String) Dim TextX As Long Dim TextY As Long TextX = Camera.Left + ((MAX_MAPX + 1) * PIC_X / 2) - getWidth(TexthDC, Trim$(text)) TextY = Camera.Bottom - 15 ' Draw information text Call DrawText(TexthDC, TextX, TextY, text, QBColor(White)) End Sub ``` (I plan on putting in the timing code after I get this solved) Any help appreciated, thanks
-
Hello, Soldier's Tale is a game I started about a month ago, I am looking for some extra help! Here is the WIP Thread: http://www.touchofdeathforums.com/smf/index.php/topic,68549.0.html To start off, I'll begin introducing myself. I'm 18, from Iowa, USA. I will be working on programming and pretty much general areas of development as project lead. I don't have any previous games that I've developed, so Soldier's Tale will be my first release. For experience, I began programming around 6 years ago and since have worked with C++, .NET languages, some web languages, and Visual Basic 6. For the game, I plan on not replicating Tibia as a whole, but using a lot of its features, so if you genuinely hate the game, then please don't post because this will create a lot of conflict. Here's the positions I'm looking for (1 person per job): **~~Content Designer~~** _this position has been occupied by lacangel19, therefor anybody wishing to contribute to game design should contact him (MSN/Email: jacksonv19 at hotmail.com)_ - designs and blueprints the game's gameplay.. like quests, NPC's, weapons, etc. Must have the ability to create balanced game designs **Programmer** - not asking for a pro vb6 programmer, but someone who knows the language along with the ins and outs of EO. Must be able to write code for new and planned features. This isn't going to be a way to make money, just a hobby, so contribute what you can when you can. You can contact me on MSN (hawkeyes_zdd_24 at hotmail.com) or here on the forums. If you're interested shoot me a message on MSN, PM me, or just reply to this post. Thanks!
-
Fabiooooooooooooooooooooo
-
here's all I could find on my hard drive here's one of me (luigi) and a bunch of friends heading to a halloween party… which turned out to be a $330 possession ticket  senior year football (#49)  at the pool (second from right)  .. and hanging with some friends (right) 
-
Thanks for the reply, always good to get help from the top
-
I'm implementing a little feature that I call Battlesight, it will be a little list box in the GUI that will display all NPC's and player's within a certain range (Tibia, Tibia, Tibia :P), so you can target them. I did some work on the server that finds players and NPC's within a range of 7 tiles and sends the information over to the client, but I was wondering if this should all be done client side? Any input appreciated, thanks
-
Haha oops- thanks for the info.. time for some serious trial and error now
-
Hey, I'm trying to find a way to have the player's name color drawn according to their current HP. Here's my code (inside Sub DrawPlayerName): ``` ' Set color of name based on amount of HP Dim hpPercent As Double hpPercent = GetPlayerVital(Index, Vitals.HP) / GetPlayerMaxVital(Index, Vitals.HP) If hpPercent > 0.75 Then color = QBColor(BrightGreen) ElseIf hpPercent > 0.5 And hpPercent 0.25 And hpPercent 0 And hpPercent