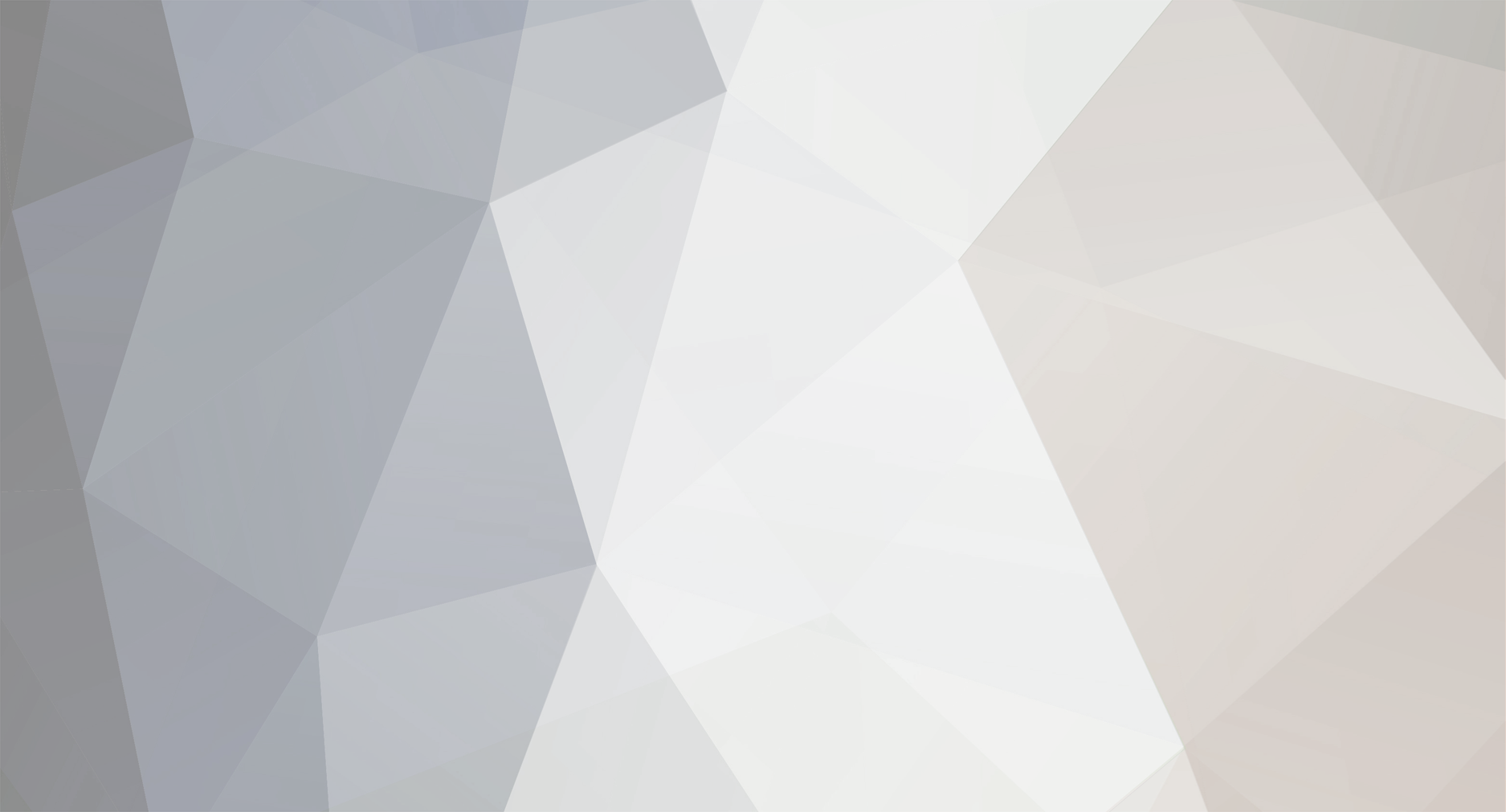
Kimimaru
Members-
Posts
890 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Everything posted by Kimimaru
-
Client does not work on Windows 7 after compiling
Kimimaru replied to silentdemonic's topic in Source
@Robin: > I've been using VB6 on Win7 64-bit for ages. Same here. The computer I'm using now has Windows 7 64-bit. I can also run Visual Basic 6 perfectly fine on my Windows 7 32-bit computer. All you really have to do is download and run the 64-bit library files here: http://eclipse-mmorpgmaker.googlecode.com/files/Eclipse%20Library%20Installer%20x86-64.exe After that, make sure you have the UAC disabled, and then run Visual Basic 6 as an administrator when you first open it up. You won't have to run it as an administrator again after that. That's all I did, and it's been running fine for me for a pretty long time. -
Hey, guys! I'm currently re-writing the timers, and I need a little help actually calling them. My main goal is to get the Server to call them from the source, not from the scripts. What I've done is made the timers their own rec, which I named TimerRec, and I've included the Name, the Interval, and the WaitTime as variables in the TimerRec. When you set a new timer, it sets the Name, Interval, and WaitTime. In Sub GameAI in the modGeneral, I have this code to handle the timers: ``` For i = 1 to 50 If GetTickCount > Timer(i).WaitTime Then If Timer(i).Name vbNullString Then Call CallByName(modGameLogic, Timer(i).Name, vbMethod) End If End If Next i ``` Now, I've read that the CallByName function doesn't work in modules, so I've tried doing the same thing with a class module, but I've still had no luck. When I put modGameLogic in the CallByName function, I either get run-time error '13': Type mismatch, or I get another error telling me that it's undefined. If I put in a class module, I get an error telling me that the class module does not support the function, or something similar to that. Anyone have any ideas? Also, if someone can provide an alternative way to doing this, I would very much appreciate it. Thanks in advance for any help!
-
@Robin: > Would you lot stop bloody bolding random pieces of text? It's really off-putting. Sorry, I only bold names of Subs, modules, forms, and some important variables so that the person I'm talking to doesn't miss them, but I'll stop. @escfoe2: > I might actually be able to figure this out lol thanks you very much.. the part about addin it at the top of ModHandleData is what really made me understand how this works.. Now I'm gonna finish my code, post it, and test it… Probably test it first but it will work or it won't so I'll post everything in a bit.. Thanks again this is a great community!!! > > OK: It isn't working yet but It's close, I have this so far: > Client-Side In ModHandleData I added a new Case: > ``` > Case "getinivar" > Call Packet_GetIniVar(Val(Parse(1)), Val(Parse(2)), Val(Parse(3))) > Exit Sub > > ```And here is the Packet_GetIniVar: > ``` > Public Sub Packet_GetIniVar(ByRef Parse() As String) > If LenB(Parse(1)) > 0 And LenB(Parse(1)) = 1 Then > If LenB(Parse(2)) > 0 Then > If LenB(Parse(3)) > 0 Then > frmStable.shpEVNT.Visible = True > frmStable.lblEVNT.Visible = True > > shpEVNT.Width = Val(Int(Parse(2)) / Int(Parse(3)) * 640) > lblEVNT.Caption = "~~ " & Int(Parse(3)) & " OUT OF " & Int(Parse(2)) & " ~~" > End If > End If > Else > frmStable.shpEVNT.Visible = False > frmStable.lblEVNT.Visible = False > End If > End Sub > > ``` Your server code is fine, but remember to replace the open quotations ("") with vbNullString. As for your Client code, you're still making mistakes with the parse() variable. Take a look at this: ``` Case "getinivar" Call Packet_GetIniVar(Val(Parse(1)), Val(Parse(2)), Val(Parse(3))) Exit Sub ``` In this code, you're already taking the values of the parses and carrying them into the Sub, so why did you pass parse() as a ByRef in your Packet_GetIniVar? You could do that if you wanted to, but you would have to pass Parse through the Sub as the only parameter, and then you would handle the individual parses (1, 2, 3) within the Sub. Instead of doing that, though, we've already handled the individual parses by passing them through Sub Packet_GetIniVar's parameters. So, all you would have to do is change your parameters in the Sub to have actual names and types. Here's how you should do it: ``` Public Sub Packet_GetIniVar(ByVal Number1 As Long, Number2 As Long, Number3 As Long) If LenB(Number1) > 0 And LenB(Number1) = 1 Then If LenB(Number2) > 0 Then If LenB(Number3) > 0 Then frmStable.shpEVNT.Visible = True frmStable.lblEVNT.Visible = True shpEVNT.Width = Val(Int(Number2) / Int(Number3) * 640) lblEVNT.Caption = "~~ " & Int(Number3) & " OUT OF " & Int(Number2) & " ~~" End If End If Else frmStable.shpEVNT.Visible = False frmStable.lblEVNT.Visible = False End If End Sub ``` Replace Number1, Number2, and Number3 with the variable names you want.
-
LenB returns the length of a variable in bytes, so you would do this: ``` Public Sub Packet_GetIniVar(ByVal Index As Long, ByVal FileName As String, ByVal Header As String, ByVal Footer As String) If LenB(FileName) > 0 And LenB(Header) > 0 And LenB(Footer) > 0 Then Call SendDataTo(Index, "GETINIVAR" & SEP_CHAR & GetVar(App.Path & FileName, Header, Footer) & SEP_CHAR & GetVar("Kill Event.ini", "KILL EVENT", "kills_needed") & SEP_CHAR & GetVar("Scripts\PlayerExtras\" & GetPlayerName(Index) & ".ini", "KILL EVENT", "kill_event_activated") & END_CHAR) End If End Sub ``` As for handling your packet data, you did correctly pass the **Parse()** variable through the Sub as **ByRef**, but you still didn't handle it correctly. I also don't know how many INI files you want to get variables from because you only sent information from one, while you tried receiving information from three. So, I'll do it with three since it seems like you wanted to do that. Try something like this: Put this at the top of **modHandleData** in the **Client** where you see all of the **Case "(packetname)"** statements: ``` Case "getinivar" Call Packet_GetIniVar(Val(parse(1)), Val(parse(2)), Val(parse(3))) Exit Sub ``` ``` Public Sub Packet_GetIniVar(ByVal KillEventActive As Long, ByVal KillEventKillsNeeded As Long, ByVal KillEventPlayerKills As Long) 'Now here it will take charge of the label and shape... shpEVNT.Width = Val(Int(KillEventKillsNeeded) / Int(KillEventPlayerKills) * 640) 'Client Side lblEVNT.Caption = "~~ " & Int(KillEventPlayerKills) & " OUT OF " & Int(KillEventKillsNeeded) & " ~~" End Sub ``` You forgot that **parses** are used to represent the variables once you've received the data from the server. **Parse(0)** is the packet name, which is already pre-handled, so all you have to do is write a new **Case** for its packet name. However, you still have to handle all of the other parses, which are all of the other parameters. I've done that when I made my call to **Packet_GetIniVar**. Plus, if you get an error saying that your label (lblEVNT) or your shape (shpEVNT) has not been declared, you have to put the form name that those variables are in before, since you're not working within that form. If they are on **frmStable**, it would be like this: ``` frmStable.shpEVNT.Width = Val(Int(KillEventKillsNeeded) / Int(KillEventPlayerKills) * 640) 'Client Side frmStable.lblEVNT.Caption = "~~ " & Int(KillEventPlayerKills) & " OUT OF " & Int(KillEventKillsNeeded) & " ~~" ``` I hope that helps!
-
@Ballie: > Better yet, use LenB and check if it's equal to 0\. ;D For some reason, when I do that with items that haven't been created yet, it returns a value of 100\. They have no name (**vbNullString**), so it's pretty strange.
-
That's fine, but instead of using open quotation marks (""), use **vbNullString**, as it will save you about 8 bytes of memory. It seems like it doesn't matter, but anything helps.
-
Think of sending packets like calling a subroutine. It's pretty similar to that, actually, in that it also has something like parameters in a way. Take this example: ``` Call PlayerMsg(Index, "Hi!", WHITE) ``` The first parameter here is the **Index**, and the second is the **Message**, which I inputted as "Hi!" The third parameter is the **color**, which I made "WHITE." Now, let's basically do the same thing by sending a packet using different separators for the parameters instead of commas: ``` Call SendDataTo(Index, "playermsg" & SEP_CHAR & Index & SEP_CHAR & "Hi!" & SEP_CHAR & WHITE & END_CHAR) ``` Here, the separators are **SEP_CHAR**. The first parameter, known as **parse(1)** when handling the data in the Client, is **Index**. I'm sure you can figure out the second and third parameters. The **END_CHAR** statement is used to tell the Client that it's the end of the packet, one of the reasons why String packets are much worse than byte arrayed packets. The **"playermsg"** refers to the packet name, which is **parse(0)** in the Client **modHandleData**. I hope that helped!
-
Oh yeah, you also have to swap the "+" signs on the Character screen in **frmStable**.
-
@Ballie: > Well, the speed/magic swap is tedious. I think you just have to change a little code in the shops, the **Client** **modHandleData**, and the inventory. I've fixed this for a couple of people before, and I don't remember it being that much code.
-
@Ballie: > I couldn't replicate this. Every time I shot a player with a bow on a safe map it says "this is a safe zone!" The problem is with hitting a player with a spell in a safe area, not hitting a player with a bow.
-
@Robin: > He can't explain it because he doesn't understand it. > > It's not his code. Maybe people looking at this topic can check out my explanation on my last post of the first page.
-
Wow, this looks great! When it gets more developed I'm definitely going to try it out! Good luck with it, Ambard!
-
You could add in a couple of fixes of my own, such as: -[Fixing Item Deletion in Shops [EE 2.7+] + Bonus Shop Fix](http://www.touchofdeathforums.com/smf/index.php/topic,53525.msg567583.html#msg567583) -[[Fix EE2.7+] Minus Stat Attribute Fix](http://www.touchofdeathforums.com/smf/index.php/topic,51801.0.html) You may have to modify the minus stat attribute fix a little bit to fit **Eclipse Stable** because of the way scripts are executed. I also have tons of other fixes that I did myself in SMBO, but I can't remember them all, unfortunately. @Robin: > Simply find anything related to IOCP and delete it, then copy across the few lines of Winsock code from Mirage. > > The Winsock code is tiny. You're using the control for crying out loud. xD Yeah, and after you implement Winsock, you can also get rid of the **clsServer**, **clsSocket**, and **colSockets** Class Modules.
-
I think this is a problem that occurs from using the 3-frame movement tutorial because after I used it, I found that my SP wasn't decreasing. The creator of that tutorial must have forgotten to add in the part where it decreases your SP when you run. You will need to edit the source code to fix this problem.
-
@Zetta: > yeah, basically. Its also a more passive means of locking than the old fps lock. And you dont really need the timer to be accurate. If you get +- 5 ms accuracy, it really wont matter. Okay, I got it. Thanks a lot!
-
I think I'll put this in my code, thanks! However, I'm still confused as to how it works. You said to make a timer and set its Interval to 66, meaning it will execute every 66 milliseconds. When it executes, according to your tutorial, it will set **CanDraw** to **True**. Then, in the main **GameLoop**, you have this code: ``` If CanDraw = False Then Exit Sub Else CanDraw = False End if ``` So basically, every 66 milliseconds, **Sub GameLoop** stops executing for another 66 milliseconds to stop processes from executing? Something similar to this is seen in the existing Eclipse Evolution system, where the program stops execution using the **Sleep** command for 1 millisecond. The loop continues until 31 milliseconds have passed by, effectively keeping the FPS down to about 31. With this system, as Robin pointed out, instead of going through the entire **GameLoop Sub** simply to lock the FPS, it does it at the beginning of the Sub so that it has to search through less code. Even with the inaccuracy of the Timer API Tool Function, this still works well? Anyway, please let me know if I'm correct on all of this. Thanks!
-
@Robin: > This tutorial is horrible though. It has absolutely no explanation and it's pretty much you just copying & pasting Fabio's code over. You claim that the tutorial is horrible, but is his edit horrible? Should I consider implementing this edit into my game?
-
There is a way to get rid of lag during night time, but you'd need to re-code the way Eclipse draws out the darkened night tiles, which we have yet to do in Super Mario Bros. Online. This also isn't easy because you need to have a pretty good knowledge on DirectX and drawing out graphics. You can make night time a little lighter pretty easily, however. Open up your **Tiles10.bmp** file and look for the colored tile to the left of the level up graphic. Change that tile's color to change the color of night time.
-
From looking at your code, I don't know if the **Pokemon1** variable is supposed to be a string or some sort of number. You should specify what type of variable it should be. Also, let's just say, for example, that **Pokemon1** is a number and that it equals **3**. The code will be looking for a file named **3.bmp** in the **Battle3** folder, which is in the **CUSTOM** folder, which is in the **GUI** folder. So, if you don't have a .bmp file named whatever **Pokemon1** is in the correct folder, then this error will come up because you don't have the file.
-
@silentdemonic: > So the Server for ES crashes after a long run time ? Ive had my server up for about 2 weeks now - 5 people logging in to edit / map / discuss constantly and no issues. Am I a rarity ? I wouldn't necessarily say a "rarity" because the way the server performs does also depend on your computer's specifications. If your computer is excellent then you will probably have fewer problems since your server will run faster.
-
For some reason, it's still not working. I've looked at the item editor, and it shows the form as vbModal yet displays the item graphics using the **Form_Load** sub. Mine won't do this for some odd reason. EDIT: I just set the **AutoRedraw** property for the Picturebox and the Form to **True**, so now it works! Thanks for the help, Robin! EDIT #2: It still doesn't work. If I make the AutoRedraw property True, it draws out the graphics when I start up the form, but it won't change them when I want it to. EDIT #3: Okay, I've finally got it! I set the **AutoRedraw** property for both the Picturebox and the Form to **True** so that it draws out the graphics when I load the form. Then, whenever I make a change in the graphics, I make it refresh the Picturebox so that it records the change. Thanks again!
-
@Robin: > You need to set BitBlt _after_ the form is loaded up. > > BitBlt is a horrible system to use anyway. It doubles your memory usage. > > Switch to BltToDC. Okay, I see, but the **Form_Load** Sub doesn't seem to be executing. I'll give it another shot with **BltToDC**. Thanks!
-
Hey, everyone! I'm just blitting out graphics in a picturebox, and it works fine whenever I change the ListIndex on my listbox in the form, but it doesn't work when the ListIndex is specified before the form is shown. Here's an example of what I'm doing: ``` Dim ItemNum As Long frmEditor.lstListBox1.ListIndex = EditorIndex ItemNum = EditorIndex + 1 Call BitBlt(frmEditor.picItems1.hDC, 0, 0, PIC_X, PIC_Y, frmMirage.picItems.hDC, (Item(ItemNum).Pic - Int(Item(ItemNum).Pic / 6) * 6) * PIC_X, Int(Item(ItemNum).Pic / 6) * PIC_Y, SRCCOPY) frmEditor.Show vbModal ``` That part is not working. However, whenever I change the ListIndex, it blits out the graphic perfectly fine. Here's an example of what I'm doing: ``` Private Sub lstListBox1_Click() Dim ItemNum As Long ItemNum = lstListBox1.ListIndex + 1 Call BitBlt(picItems1.hDC, 0, 0, PIC_X, PIC_Y, frmMirage.picItems.hDC, (Item(ItemNum).Pic - Int(Item(ItemNum).Pic / 6) * 6) * PIC_X, Int(Item(ItemNum).Pic / 6) * PIC_Y, SRCCOPY) End Sub ``` The strange thing is that this part works, while the other part doesn't draw out the graphics. Can someone please explain why this is happening?
-
Oh, okay. Thanks for telling me that.