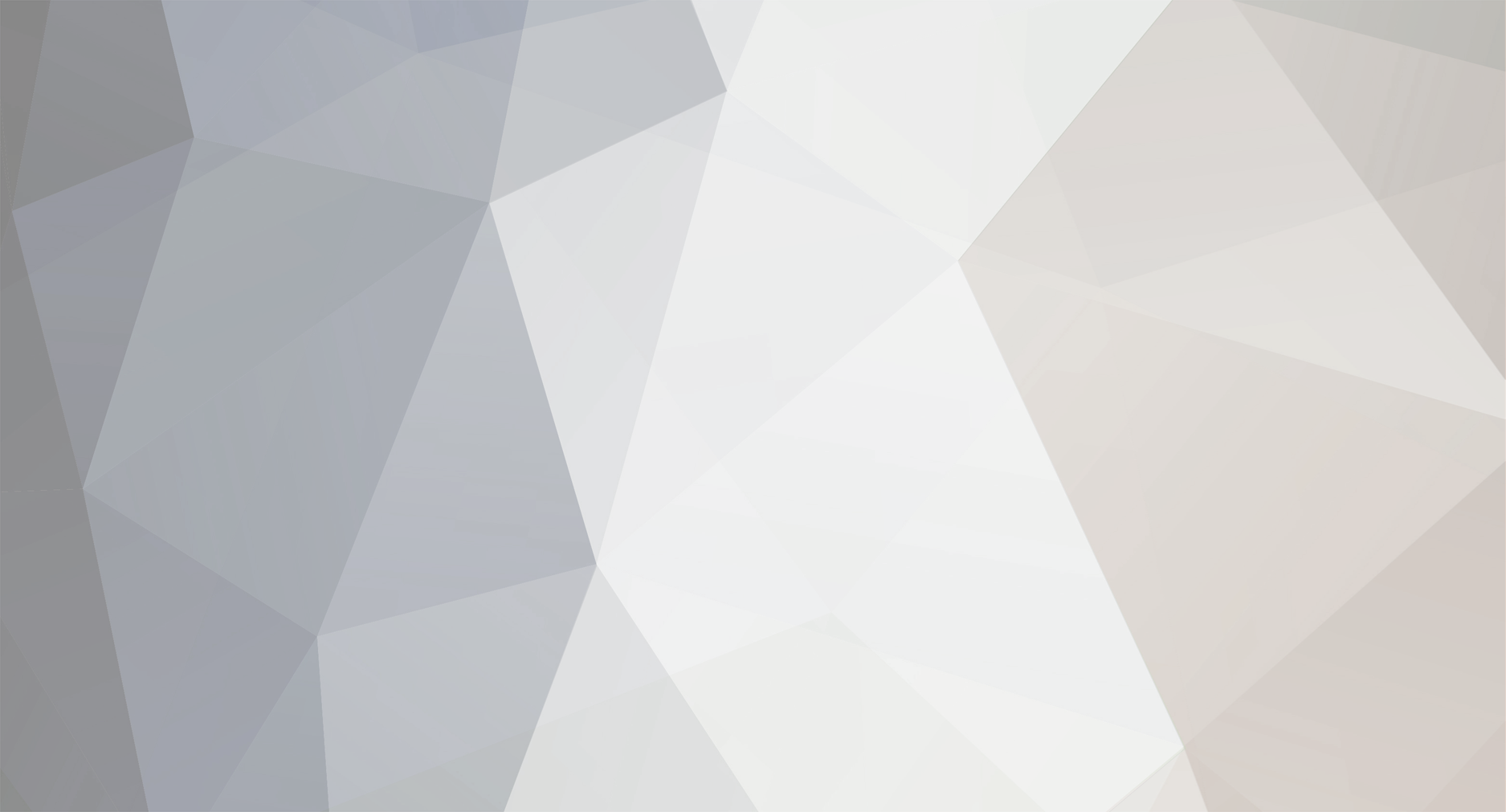
00GuthixLord
-
Posts
154 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Posts posted by 00GuthixLord
-
-
1,000,000 - 90841 = 9159
-
-
-
I think the Hp,MP, And EXP bars on the second one are to light
-
Ok it could of been my fault i was getting the error because:
```
If n <> index Then
End If
```
Checks to see if i'm healing someone else and I wan't so it didn't do any thing… Oops :embarrassed:
Well at least i finished my button :)
Edit: @Joost:
> ```
> ' Restore vitals
> Call SetPlayerVital(index, Vitals.HP, GetPlayerMaxVital(index, Vitals.HP))
> Call SetPlayerVital(index, Vitals.MP, GetPlayerMaxVital(index, Vitals.MP))
> Call SendVital(index, Vitals.HP)
> Call SendVital(index, Vitals.MP)
> ' send vitals to party if in one
> If TempPlayer(index).inParty > 0 Then SendPartyVitals TempPlayer(index).inParty, index
>
> ```How about that?
That Code Was The Only One That Worked Though -
-
-
-
Ok so I'm working on one of my codes then i need to full heal and i change my code like 100 times and nothing works here is what i'm using for my code:
```
' The player
n = FindPlayer(buffer.ReadString) 'Parse(1))
epic = 10000
Set buffer = Nothing
If n <> index Then
If n > 0 Then
Player(n).Vital(1) = Player(n).Vital(1) + epic
Call PlayerMsg(n, "You Feel Refreshed.", BrightBlue)
Call AddLog("You Healed" & GetPlayerName(n) & ".", ADMIN_LOG)
Else
Call PlayerMsg(index, "Player is not online.", White)
End If
End If
```
Some of the code was me just trying different things but the code that's a problem is:
```
Player(n).Vital(1) = Player(n).Vital(1) + epic
```So How Would I Change That So It Would Heal Them Fully? -
-
-
-
-
Well I'm working on the graphics on my RPG and I don't know how good I'm doing so i finished the main menu so far and here it is:
Main:
>! 
Newest:
>! Menu:

Equipment Tab:

Note: It's Not Labeled Yet…
Almost Complete Try 1:
>! 
Try 3:
>! 
Try 2:
>! 
Try 1:
>! 
Buttons Finished:
Login
Register
Credits
Exit -
@Lenn:
> If you actually read the code, you would find it clearly draws text with the GDI text rendering system. Not exactly the rendering of the bitmap font that D3D8 offers, is it?
I'm new to this and i just use the one on the download link so…It's the event system one. I don't know any other ones... -
Oh i didn't see that there was another tutorial for it, I looked for a while for one, And ill try to space it out more. The hardest part is the caps, I either put caps at every letter or i don't it's just a habit. So thanks :)
LoL this took a while to write getting the caps and stuff… -
I Couldn't Find A Tutorial For This So I Went In The Code, Messed Around And I Got It To Work :)
Level Outcome:

This Is All Client Based, It's All In modText, And The Sub DrawPlayerName.
Ok First We Need To Add The Variables We Are Going To Use. So Find This:
```
Dim TextX As Long
Dim TextY As Long
Dim color As Long
Dim Name As String
```And Add These To The List:
```
Dim TextY2 As Long
Dim Level As String
```Those Will Be Explained Soon. Now If You Go Down And Find This:
```
Name = Trim$(Player(Index).Name)
```I Copied & Pasted That Code Under It But Changed It To This:
```
Level = Trim$(Player(Index).Level)
```That Tells The Code That The Variable Level Is The Players Level. Now About 3 Lines Under That Is This:
```
TextY = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - 16
```Copy And Paste That Under It, Then Change It So It Looks Like This:
```
TextY2 = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - 16
```That Tells The Code Where TextY2 is Located On The Player. Now You See That They Both Say 16\. Well That's Bad, They Will Both Be Touching Like That, So I Changed The TextY's 16 To -1 And I Thought That It Looked Good, But If You Don't Like It Then You Can Change It.
Now About 3 Lines Under Is This:
```
TextY = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - (DDSD_Character(GetPlayerSprite(Index)).lHeight / 4) + 16
```Ok, You Need To Copy And Paste That Under It. Before You Edit The One You Pasted Change The 16 On The First One To -1\. Now Just Add The 2 On The Pasted One So It Looks Like This:
```
TextY2 = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - (DDSD_Character(GetPlayerSprite(Index)).lHeight / 4) + 16
```Now A Couple Lines Down Is This:
```
Call DrawText(TexthDC, TextX, TextY, Name, color)
```Now Again I Would Copy Then Paste Under It. Change The Bottom One To This:
```
Call DrawText(TexthDC, TextX - 1, TextY2, "[Lv. " & Level & "]", color)
```That Tells The Code To Position It -1 Off The Normal(So It's Centered) And Adds The [Lv. 1] Under The Name. If The -1 Isn't Centered You Can Change It. If Some One Has A Way To Easily Center It Better I Would Like To Know Thanks. :cheesy:
I Hope This Works For You And Helps :cheesy:
Finished Code
>! Variables:
```
Dim TextX As Long
Dim TextY As Long
Dim TextY2 As Long
Dim color As Long
Dim Name As String
Dim Level As String
>! ```The Other Stuff:
```
Name = Trim$(Player(Index).Name)
Level = Trim$(Player(Index).Level)
' calc pos
TextX = ConvertMapX(GetPlayerX(Index) * PIC_X) + Player(Index).XOffset + (PIC_X \ 2) - getWidth(TexthDC, (Trim$(Name)))
If GetPlayerSprite(Index) < 1 Or GetPlayerSprite(Index) > NumCharacters Then
TextY = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - -1
TextY2 = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - 16
Else
' Determine location for text
TextY = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - (DDSD_Character(GetPlayerSprite(Index)).lHeight / 4) + -1
TextY2 = ConvertMapY(GetPlayerY(Index) * PIC_Y) + Player(Index).YOffset - (DDSD_Character(GetPlayerSprite(Index)).lHeight / 4) + 16
End If
>! ' Draw name
Call DrawText(TexthDC, TextX, TextY, Name, color)
Call DrawText(TexthDC, TextX - 1, TextY2, "[Lv. " & Level & "]", color)
>! ```
Sorry If I Messed Up On Grammar Or Spelling I'm Bad A Typing Tutorials, So I Tend To Mess Up. ;) -
MSDN error
in Q & A
Thanks Now I Can Start Editing The Code :cheesy: -
MSDN error
in Q & A
I Reinstalled VB6 And I Still Get The Error -
MSDN error
in Q & A
ok -
MSDN error
in Q & A
How Do I Reinstall? -
I Just Got It I Wanted To See What It Was :)
-
MSDN error
in Q & A
Ok If This Is In Wrong Section Please Move.
Ok I Open VB6 Client And I Get An Error That Says:
Errors During Load. Refer to frmEditor_Events.log For Details.
Then When I Hit Help it Says The MSDN collection does not exist. Please Reinstall MSDN.
So What Is MSDN? -
wow i really want to try this!
Looks Real Cool
[EO - Event System] Heal Player From Admin Panel
in Source
Posted
* * *
Outcome:
>! 
* * *
Client:
Ok for to start off we need a button:
* Open client
* Then frmMain
* Expand frmMain To The Right
* Rearrange the admin panel so you can fit in another button
* After you add in your button double click and it will take you to some code
The code should look something like this:
```
Private Sub HealPlayer_Click()
End Sub
```
The first thing we should add is the debug so its a simple code that looks like this:
```
' If debug mode, handle error then exit out
If Options.Debug = 1 Then On Error GoTo errorhandler
Exit Sub
errorhandler:
HandleError "HealPlayer_Click", "frmMain", Err.Number, Err.Description, Err.Source, Err.HelpContext
Err.Clear
Exit Sub
```
Now to prevent hackers add:
```
If GetPlayerAccess(MyIndex) < ADMIN_MAPPER Then
Exit Sub
End If
```That checks if you the owner/admin. Now we need it to do some thing so make a variable name, I chose HealThem so I would add this:
```
HealThem Trim$(txtAName.text)
```That tells the code to read from some where else, Now open modClientTCP.
In there find a empty spot that is not in a sub and make your own sub. It should look something like this(With The Debug):
```
Public Sub HealThem(ByVal Name As String)
' If debug mode, handle error then exit out
If Options.Debug = 1 Then On Error GoTo errorhandler
Exit Sub
errorhandler:
HandleError "HealThem", "modClientTCP", Err.Number, Err.Description, Err.Source, Err.HelpContext
Err.Clear
Exit Sub
End Sub
```Ok now that we have are sub we need to tell it what to do so add this:
```
' This goes under your sub name
Dim Buffer As clsBuffer
'This Goes After The Goto Errorhandler
Set Buffer = New clsBuffer
Buffer.WriteLong CHealer
Buffer.WriteString Name
SendData Buffer.ToArray()
Set Buffer = Nothing
```That tells the code to send the name to the server with CHealer you can change that but make sure it has a C in front.
Ok to make it so the server receives that you need to open modEnumerations and find this:
```
' Packets sent by client to server
Public Enum ClientPackets
```There is a big long list after it, you need to add CHealer after the last 'C' thing But Before CMSG_COUNT .
Example:
>! ```
CDeclineTradeRequest
CPartyRequest
CAcceptParty
CDeclineParty
CPartyLeave
CEventChatReply
CEvent
CSwitchesAndVariables
CRequestSwitchesAndVariables
CHealer ' Its After The Last C thing
>! ```
* * *
Server:
Ok your in the server now so open modEnumerations first and add the last part of Client in the same spot.
Ok after that you need to open modHandleData, and find a nice open spot and add this:
```
Sub HandleHealThem(ByVal index As Long, ByRef Data() As Byte, ByVal StartAddr As Long, ByVal ExtraVar As Long, ByVal Vital As Vitals)
Dim n As Long
Dim buffer As clsBuffer
Set buffer = New clsBuffer
buffer.WriteBytes Data()
' Prevent hacking
If GetPlayerAccess(index) < ADMIN_MAPPER Then
Exit Sub
End If
' The player
n = FindPlayer(buffer.ReadString) 'Parse(1))
Set buffer = Nothing
If n <> index Then
If n > 0 Then
Else
End If
Else
End If
End Sub
```Explanation:
>! ```
Sub HandleHealThem(ByVal index As Long, ByRef Data() As Byte, ByVal StartAddr As Long, ByVal ExtraVar As Long, ByVal Vital As Vitals)
>! ```That starts the sub with all your values you need.
The HandleHealThem change that to the Variable you created for the Trim$ part in the client.
>! ```
Dim n As Long
Dim buffer As clsBuffer
Set buffer = New clsBuffer
buffer.WriteBytes Data()
```That creates your variable n, and Buffer then sets buffer as the clsBuffer and gets your data you sent from client.
>! ```
' Prevent hacking
If GetPlayerAccess(index) < ADMIN_MAPPER Then
Exit Sub
End If
>! ' The player
n = FindPlayer(buffer.ReadString) 'Parse(1))
Set buffer = Nothing
>! ```These kinda explain them self's, but the set buffer just stops the buffer from working because it has all the data it needs.
>! ```
If n <> index Then
If n > 0 Then
Else
End If
Else
End If
>! ```That checks to see if your using it on your self or another person.. The 'If n > 0 Then' checks if the player is on.
Now to add healing replace the whole 'If n <> index then' statement with this:
```
If n <> index Then
If n > 0 Then
Call SetPlayerVital(n, Vitals.HP, GetPlayerMaxVital(n, Vitals.HP))
Call SetPlayerVital(n, Vitals.MP, GetPlayerMaxVital(n, Vitals.MP))
Call SendVital(n, Vitals.HP)
Call SendVital(n, Vitals.MP)
Call PlayerMsg(n, "You Feel Refreshed.", BrightBlue)
Call AddLog("You Healed" & GetPlayerName(n) & ".", ADMIN_LOG)
Else
Call PlayerMsg(index, "Player is not online.", White)
End If
Else
Call SetPlayerVital(n, Vitals.HP, GetPlayerMaxVital(n, Vitals.HP))
Call SetPlayerVital(n, Vitals.MP, GetPlayerMaxVital(n, Vitals.MP))
Call SendVital(n, Vitals.HP)
Call SendVital(n, Vitals.MP)
Call PlayerMsg(n, "You Feel Refreshed.", BrightBlue)
Call AddLog("You Healed" & GetPlayerName(n) & ".", ADMIN_LOG)
End If
```
Explanation:
>! ```
If n > 0 Then
Call SetPlayerVital(n, Vitals.HP, GetPlayerMaxVital(n, Vitals.HP))
Call SetPlayerVital(n, Vitals.MP, GetPlayerMaxVital(n, Vitals.MP))
Call SendVital(n, Vitals.HP)
Call SendVital(n, Vitals.MP)
Call PlayerMsg(n, "You Feel Refreshed.", BrightBlue)
Call AddLog("You Healed" & GetPlayerName(n) & ".", ADMIN_LOG)
Else
Call PlayerMsg(index, "Player is not online.", White)
End If
```That gets a player that wasn't a the person using the button and heals them.
```
The 'If n > 0 Then' Checks If There Online
Else
Call SetPlayerVital(n, Vitals.HP, GetPlayerMaxVital(n, Vitals.HP))
Call SetPlayerVital(n, Vitals.MP, GetPlayerMaxVital(n, Vitals.MP))
Call SendVital(n, Vitals.HP)
Call SendVital(n, Vitals.MP)
Call PlayerMsg(n, "You Feel Refreshed.", BrightBlue)
Call AddLog("You Healed" & GetPlayerName(n) & ".", ADMIN_LOG)
End If
```The Else Say's That its the Admin Healing Them Self And Heals Them.
>! ```
Call SetPlayerVital(n, Vitals.HP, GetPlayerMaxVital(n, Vitals.HP))
Call SetPlayerVital(n, Vitals.MP, GetPlayerMaxVital(n, Vitals.MP))
```That Gets The Players Max HP and MP Then:
```
Call SendVital(n, Vitals.HP)
Call SendVital(n, Vitals.MP)
```That Gives Them There Max HP And MP
```
Call PlayerMsg(n, "You Feel Refreshed.", BrightBlue)
```That Just Tells The Player He Was Healed
>! ```
Call AddLog("You Healed" & GetPlayerName(n) & ".", ADMIN_LOG)
```That Puts It Into The Server Log.
I Hope That Helped. And I Put Enough Explanation In It. :P
Sorry If There Are Any Misspellings Or Grammar Mishaps Writing Tutorials With All The Spoilers And Code Makes Me Mess Up Alot. :embarrassed: