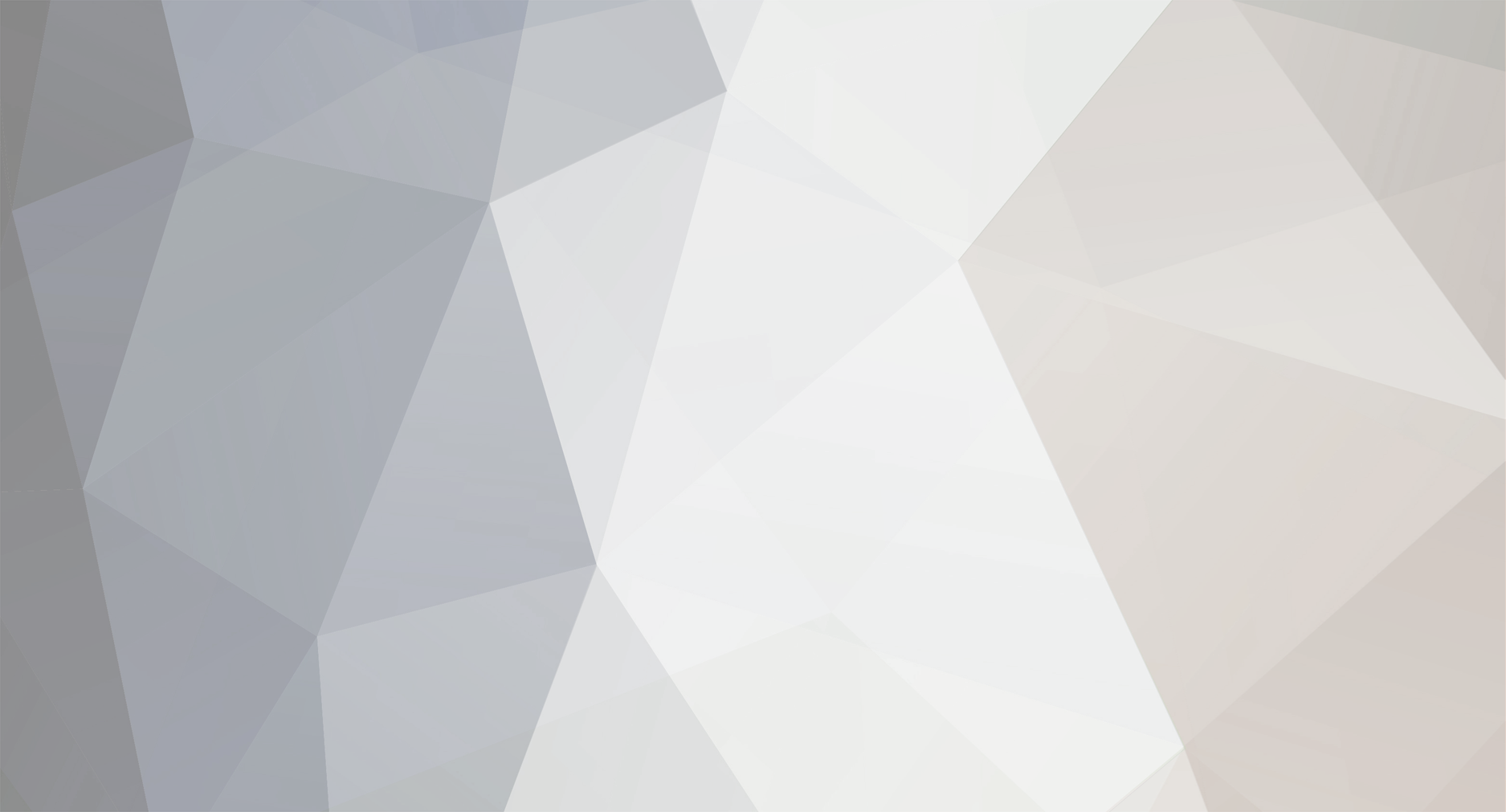
viciousdead
Members-
Posts
990 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Everything posted by viciousdead
-
This is a very simple code that allows you to change the experiance(exp) rate of your game without going through and editting npc's. If I get the time, I'll add more. **Everything Is Server-sided** **First make a scroll bar on the Server Conrol Panel and name it "scrlExpRate". Then above it make a label and call it "lblExpRate", should look something like this:** >!  **Now double click the scroll bar, and paste in this code:** >! ``` Private Sub scrlExpRate_Change() Dim EXPRATE As Long >! lblExpRate.Caption = "Exp Rate: " & frmServer.scrlExpRate.Value EXPRATE = frmServer.scrlExpRate.Value If frmServer.scrlExpRate.Value > 1 Then Call GlobalMsg("Experiance Rate For The Server Is Currently: " & frmServer.scrlExpRate.Value & "x", Yellow) Else If frmServer.scrlExpRate.Value = 0 Then Call GlobalMsg("Experiance Rate For The Server Is Currently: Frozen", Yellow) Else Call GlobalMsg("Experiance Rate For The Server Is Currently: Normal", Yellow) End If End If End Sub ``` **Now go to modCombat, then search for "Sub PlayerAttackNpc", add this code underneath it:** >! ``` Dim EXPRATE As Long ```**Last, in modCombat, search for "Calculate exp to give attacker", replace that code with:** >! ``` ' Check server's experaince rate EXPRATE = frmServer.scrlExpRate.Value ' Calculate exp to give attacker exp = (Npc(npcNum).exp * EXPRATE) ``` **Screenshots** >! [](http://www.freemmorpgmaker.com/files/imagehost/#db8394a49f6866bc495c65dbc66bd220.png) [](http://www.freemmorpgmaker.com/files/imagehost/#4b87bfd97134de0bba790dd1fd335ca7.png)
-
@Yukiyo: > Go to where it handles the experience for the player and manipulate the algorithm. That's the best I can help. > > Also: Ctrl + F Found out what was wrong…. The sequence in which I defined the variables.. XD Fail!~
-
@Yukiyo: > Eh, just edit modCombat where it handles the exp and exp roll over. Add the ratechange to the algorithm. Like I said, no modCombat.
-
@Yukiyo: > It's different in that version, kind of. xP I suggest upgrading to EO v2b and reading this: [[EO] Packet Tutorial](http://www.touchofdeathforums.com/smf/index.php/topic,69393.0.html) Ok, I read that, but I still don't get why we have to Send a packet to make this work… It's not Client-sided in any way, its all Server Sided... Scroll bar code for frmserver: >! ``` Private Sub ScrlEXP_Change() Dim EXPRATE As Long If ScrlEXP.Value > 0 Then EXPRATE = ScrlEXP.Value lblEXP.Caption = "Exp Rate: " & ScrlEXP.Value Else EXPRATE = 0 lblEXP.Caption = "Exp Rate: Zero" End If End Sub >! ``` Here is my modplayer script: >! ``` Sub AttackPlayer(ByVal Attacker As Long, ByVal Victim As Long, ByVal Damage As Long, Optional ByVal spellnum As Long = 0) Dim exp As Long Dim killxp As Long Dim EXPTOTAL As Long Dim EXPRATE As Long Dim n As Long Dim i As Long Dim Buffer As clsBuffer >! ' Check for subscript out of range If IsPlaying(Attacker) = False Or IsPlaying(Victim) = False Or Damage < 0 Then Exit Sub End If >! ' Check for weapon n = 0 >! If GetPlayerEquipment(Attacker, Weapon) > 0 Then n = GetPlayerEquipment(Attacker, Weapon) End If >! ' Send this packet so they can see the person attacking Set Buffer = New clsBuffer Buffer.WriteLong SAttack Buffer.WriteLong Attacker SendDataToMapBut Attacker, GetPlayerMap(Attacker), Buffer.ToArray() Set Buffer = Nothing >! If Damage >= GetPlayerVital(Victim, Vitals.HP) Then 'Call PlayerMsg(Attacker, "You hit " & GetPlayerName(Victim) & " for " & Damage & " hit points.", White) 'Call PlayerMsg(Victim, GetPlayerName(Attacker) & " hit you for " & Damage & " hit points.", BrightRed) SendActionMsg GetPlayerMap(Victim), "-" & Damage, BrightRed, 1, (GetPlayerX(Victim) * 32), (GetPlayerY(Victim) * 32) ' Calculate exp to give attacker exp = (GetPlayerExp(Victim) \ 10) ' Calculate exp of a kill killxp = (GetPlayerLevel(Victim) * EXPTOTAL) EXPTOTAL = (EXPRATE * 100) EXPRATE = frmServer.ScrlEXP.Value >! ' Make sure we dont get less then 0 If exp < 0 Then exp = 0 End If >! If exp = 0 Then Call PlayerMsg(Victim, "You lost no exp.", BrightRed) Call PlayerMsg(Attacker, "You received " & killxp & " exp for killing " & GetPlayerName(Victim) & ".", BrightBlue) Call SetPlayerExp(Attacker, GetPlayerExp(Attacker) + killxp) SendEXP Attacker Else Call SetPlayerExp(Victim, GetPlayerExp(Victim) - exp) SendEXP Victim Call PlayerMsg(Victim, GetPlayerName(Attacker) & " absorbed " & exp & " exp from you.", BrightRed) Call SetPlayerExp(Attacker, GetPlayerExp(Attacker) + killxp) Call SetPlayerExp(Attacker, GetPlayerExp(Attacker) + exp) SendEXP Attacker Call PlayerMsg(Attacker, "You received " & killxp & " exp for killing " & GetPlayerName(Victim) & ".", BrightBlue) Call PlayerMsg(Attacker, "You absorbed " & exp & " bonus exp from " & GetPlayerName(Victim) & ".", BrightGreen) End If >! ' Check for a level up Call CheckPlayerLevelUp(Attacker) >! ' Check if target is player who died and if so set target to 0 If TempPlayer(Attacker).TargetType = TARGET_TYPE_PLAYER Then If TempPlayer(Attacker).Target = Victim Then TempPlayer(Attacker).Target = 0 TempPlayer(Attacker).TargetType = TARGET_TYPE_NONE End If End If >! Call OnDeath(Victim) Else ' Player not dead, just do the damage Call SetPlayerVital(Victim, Vitals.HP, GetPlayerVital(Victim, Vitals.HP) - Damage) Call SendVital(Victim, Vitals.HP) SendActionMsg GetPlayerMap(Victim), "-" & Damage, BrightRed, 1, (GetPlayerX(Victim) * 32), (GetPlayerY(Victim) * 32) 'if a stunning spell, stun the player If spellnum > 0 Then If Spell(spellnum).StunDuration > 0 Then StunPlayer Victim, spellnum End If End If >! ' Reset attack timer TempPlayer(Attacker).AttackTimer = GetTickCount End Sub ``` Those are the ONLY scripts/codes I have changed, this is just dealing with the exp rate of pvp.
-
Ugh… Any idea on what I need to send...? I really dont know that much about sending/receiving packets... :(
-
@Yukiyo: > On the event where you change the scroll bar, send a packet to the client with it's value and have the client handle that value, and modify a global expratechange variable with it, which can be used in modCombat. modCombat? I'm using the EO Side-scrolling client by MrMiguu… Outdated maybe?
-
@Captain: > I'm not really understanding your question.. > > Rewrite the whole post again without all the crosses. > > Edit > > To Define exprate you would put > > Dim Exprate as long > > Long being the type of variable. I know that, I need to know how to update/refresh the exp rate, so that it reflects in-game… :o
-
Ok, I'm horrible at explaining things. On my Server Control Panel, I have a scroll bar that changes the exp rate of PvP kills, the equation is like this: killxp = GetPlayerLevel(Victim)(Exp Rate * 100) killxp is the amount of experiance total you will receive for that kill. I have everything working except, the fact that when I change the Exp Rate on my Server Control Panel, the experience earned in-game doesn't *update*, it stays at 0.. 
-
~~Ok, so I have ScrlEXPRATE.Value = EXPRATE and in another piece of code I have an equation(this just being part of it): EXPTOTAL = (EXPRATE * 100) How do I define EXPRATE or w/e, all of this is server-sided.. I'm a bit confused. :confused: I get confused on the easy stuff guys, so over look me and don't be a t-bag. :embarrassed:~~ Currently the equation is like this: killxp = (GetPlayerLevel(Victim) * EXPTOTAL) EXPTOTAL = (EXPRATE * 100) EXPRATE = frmServer.ScrlEXP.Value the scrlEXP.value is 0 so 0*100= 0 right? Well I changed the 0 on the server control panel to like 10, and killed another person 10*100 = 1000 but it still gave me 0, how do I make it update the exp rate when I raise/lower it?
-
@MrMiguu: > It's not hard at all to make. I agree with you on the buffs suggestion. Those are very general for most games. > > For the record: I really hate seeing other programmers tell people, reporting **actual bugs** in the EO Bugs thread, that they can easily fix those bugs themselves. If its a bug, it shouldn't be fixed by the developer; it should be fixed by the engine developer. Agreed.
-
Fable 2, why you ask? Because you can get married to multiply people, then have them meet and they'll have a b!tch fight! :cheesy:
-
@Rainbow: > I'm hard.
-
Simple bug: >! Party box overlaps admin panel. o.e EDIT: Oh, same thing above me. ;)
-
Does EO support .wav yet?
-
> "Apparently you can set people on fire just by thinking about it. You would question the origins of such a skill and perhaps think about the possible side-effects, but fuck it. You can set people on fire." > "The origins of this axe are unknown, but whenever you hit someone across the face with it horrible Japanese tentacles sprout from the ground and violate your victim." Robin, I love your creative imagination, lol'd for like 15 minutes.
-
Has anyone played around with Visual Studio 2010?
viciousdead replied to viciousdead's topic in Discussion
@Robin: > IF YOU'RE ASKING THAT QUESTION THEN YOU DON'T KNOW ENOUGH ABOUT THE MECHANICS OF PROGRAMMING TO EVEN BEGIN WRITING YOUR OWN ENGINE. ilu to -
Has anyone played around with Visual Studio 2010?
viciousdead replied to viciousdead's topic in Discussion
@Robin: > Program your own engine or use netGore or something? Eclipse is written in VB6\. VB.NET is completely unrelated. Well, I guess this is an unrelated program, but the post was a question from me involving eclipse. @Soul: > I don't think you catch my drift. You would have to rewrite the engine to make Eclipse work in VS10. THATS WHAT I WAS WANTING TO KNOW SO ITS POSSIBLE? :D -
Has anyone played around with Visual Studio 2010?
viciousdead replied to viciousdead's topic in Discussion
@Soul: > That's what I meant. Dam it now I'm confused, what can you do with VS'10? -
Has anyone played around with Visual Studio 2010?
viciousdead replied to viciousdead's topic in Discussion
@Soul: > You can't program in VS. That's .NET. Only Visual Basic 6 Professional or Enterprise will work. (There is a convert option, but it produces thousands of bugs.) What do you mean you can't program in VS? That's the reason it was made… To program/create window apps. -
Has anyone played around with Visual Studio 2010?
viciousdead replied to viciousdead's topic in Discussion
@Robin: > 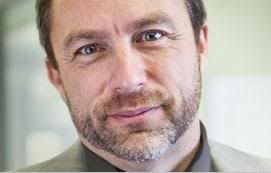 Robin I don't understand, prehaps you should use a picture of sarah palin to descibe what you feel. -
Has anyone played around with Visual Studio 2010?
viciousdead replied to viciousdead's topic in Discussion
Is this a bad way to program an eclipse game? I am downloading VS2010 to try it out, it looks like VB6. :confused: -
Does this edition of EO support png format, because jpeg is stupid.
-
@Marsh: > Dude I can grab weed out of my pocket grab my pipe pack it full of weed and smoke it all without getting off the longboard while skating. Pro.
-
If you see naked people, you may be an exlipse addict.
-
Client-Sideded GetPlayerLevel Function doesn't work for me.. :icon_alabanza: