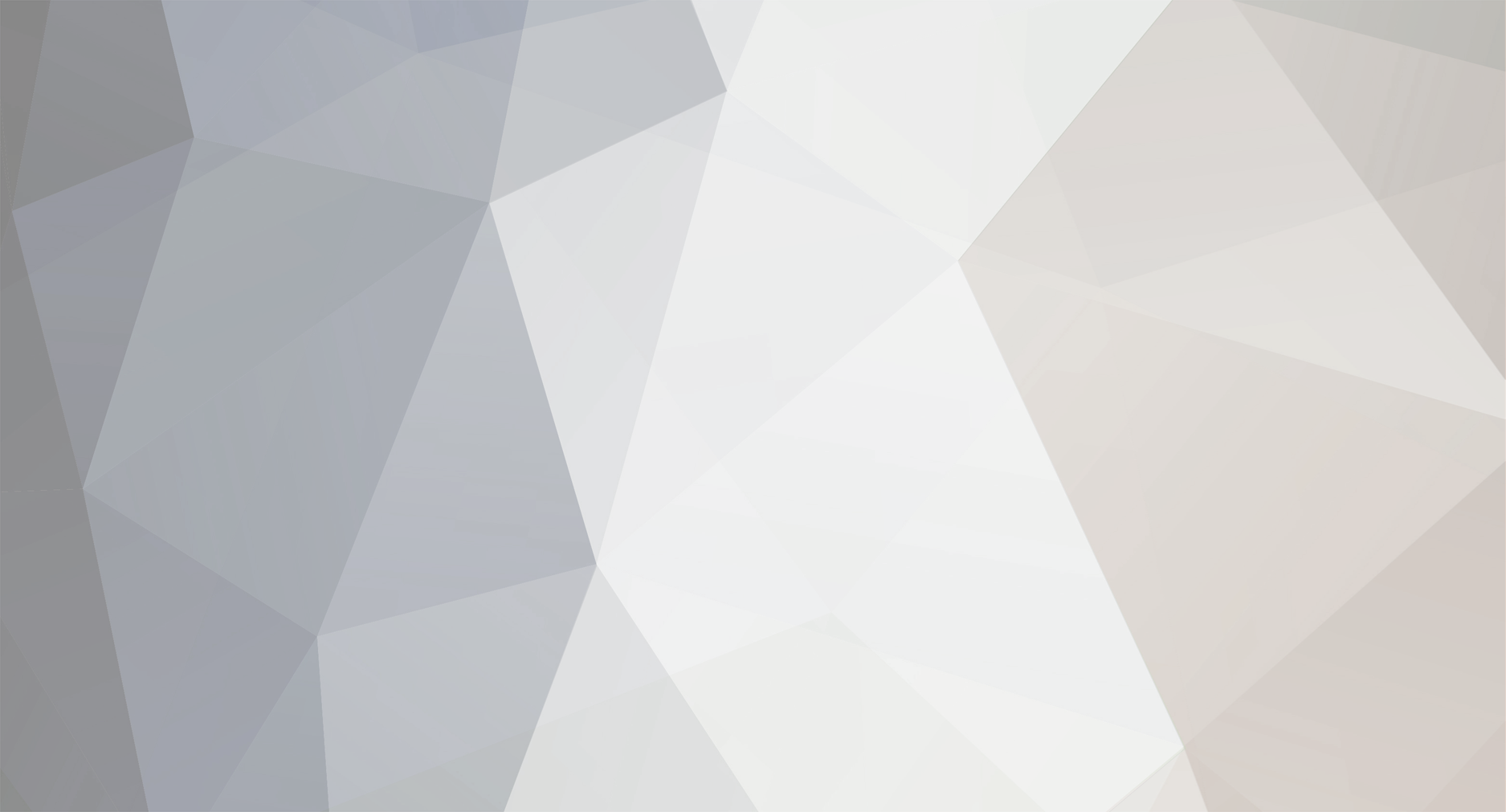
Doraemon
-
Posts
63 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Posts posted by Doraemon
-
-
(bump for help)
anyone? -
```
Private Sub InitSurfaces()
Dim rec As DxVBLib.RECT
' If debug mode, handle error then exit out
If Options.Debug = 1 Then On Error GoTo errorhandler
' DirectDraw Surface memory management setting
DDSD_Temp.lFlags = DDSD_CAPS
DDSD_Temp.ddsCaps.lCaps = DDSCAPS_OFFSCREENPLAIN Or DDSCAPS_SYSTEMMEMORY
' clear out everything for re-init
Set DDS_BackBuffer = Nothing
' Initialize back buffer
With DDSD_BackBuffer
.lFlags = DDSD_CAPS Or DDSD_WIDTH Or DDSD_HEIGHT
.ddsCaps.lCaps = DDSD_Temp.ddsCaps.lCaps
.lWidth = (MAX_MAPX + 3) * PIC_X
.lHeight = (MAX_MAPY + 3) * PIC_Y
End With
Set DDS_BackBuffer = DD.CreateSurface(DDSD_BackBuffer)
' load persistent surfaces
If FileExist(App.Path & "\data files\graphics\door.bmp", True) Then Call InitDDSurf("door", DDSD_Door, DDS_Door)
If FileExist(App.Path & "\data files\graphics\direction.bmp", True) Then Call InitDDSurf("direction", DDSD_Direction, DDS_Direction)
If FileExist(App.Path & "\data files\graphics\target.bmp", True) Then Call InitDDSurf("target", DDSD_Target, DDS_Target)
If FileExist(App.Path & "\data files\graphics\misc.bmp", True) Then Call InitDDSurf("misc", DDSD_Misc, DDS_Misc)
If FileExist(App.Path & "\data files\graphics\blood.bmp", True) Then Call InitDDSurf("blood", DDSD_Blood, DDS_Blood)
If FileExist(App.Path & "\data files\graphics\bars.bmp", True) Then Call InitDDSurf("bars", DDSD_Bars, DDS_Bars)
If FileExist(App.Path & "\data files\graphics\gui\main\hud.bmp", True) Then Call InitDDSurf("gui\main\hud", DDSD_HUD, DDS_HUD)
' count the blood sprites
BloodCount = DDSD_Blood.lWidth / 32
' Error handler
Exit Sub
errorhandler:
HandleError "InitSurfaces", "modDirectDraw7", Err.Number, Err.Description, Err.Source, Err.HelpContext
Err.Clear
Exit Sub
End Sub
```
```
Public Sub BltHUD()
Dim x As Long, y As Long, rec As DxVBLib.RECT
'Rect/Rec is a simple way to say Rectangle. The RECT is the area to grab from your bmp
'In this case it is the whole thing while in sprites/tiles it is only portions.
With rec
.top = 0
.Left = 0
.Right = 0
.Bottom = 0
End With
'V This here basically tells it to cut out ur color in pixel 1x1.
Call Engine_BltFast(Camera.Right - rec.Right, Camera.top, DDS_HUD, rec, DDBLTFAST_SRCCOLORKEY)
End Sub
```
```
Public Sub Render_Graphics()
Dim x As Long
Dim y As Long
Dim i As Long
Dim rec As DxVBLib.RECT
Dim rec_pos As DxVBLib.RECT
' If debug mode, handle error then exit out
If Options.Debug = 1 Then On Error GoTo errorhandler
' check if automation is screwed
If Not CheckSurfaces Then
' exit out and let them know we need to re-init
ReInitSurfaces = True
Exit Sub
Else
' if we need to fix the surfaces then do so
If ReInitSurfaces Then
ReInitSurfaces = False
ReInitDD
End If
End If
' don't render
If frmMain.WindowState = vbMinimized Then Exit Sub
If GettingMap Then Exit Sub
' update the viewpoint
UpdateCamera
' update animation editor
If Editor = EDITOR_ANIMATION Then
EditorAnim_BltAnim
End If
' fill it with black
DDS_BackBuffer.BltColorFill rec_pos, 0
BltHUD
' blit lower tiles
If NumTileSets > 0 Then
For x = TileView.Left To TileView.Right
For y = TileView.top To TileView.Bottom
If IsValidMapPoint(x, y) Then
Call BltMapTile(x, y)
End If
Next
Next
End If
' render the decals
For i = 1 To MAX_BYTE
Call BltBlood(i)
Next
' Blit out the items
If NumItems > 0 Then
For i = 1 To MAX_MAP_ITEMS
If MapItem(i).num > 0 Then
Call BltItem(i)
End If
Next
End If
' draw animations
If NumAnimations > 0 Then
For i = 1 To MAX_BYTE
If AnimInstance(i).Used(0) Then
BltAnimation i, 0
End If
Next
End If
' Y-based render. Renders Players, Npcs and Resources based on Y-axis.
For y = 0 To Map.MaxY
If NumCharacters > 0 Then
' Players
For i = 1 To Player_HighIndex
If IsPlaying(i) And GetPlayerMap(i) = GetPlayerMap(MyIndex) Then
If Player(i).y = y Then
Call BltPlayer(i)
End If
End If
Next
' Npcs
For i = 1 To Npc_HighIndex
If MapNpc(i).y = y Then
Call BltNpc(i)
End If
Next
End If
' Resources
If NumResources > 0 Then
If Resources_Init Then
If Resource_Index > 0 Then
For i = 1 To Resource_Index
If MapResource(i).y = y Then
Call BltMapResource(i)
End If
Next
End If
End If
End If
Next
' animations
If NumAnimations > 0 Then
For i = 1 To MAX_BYTE
If AnimInstance(i).Used(1) Then
BltAnimation i, 1
End If
Next
End If
' blit out upper tiles
If NumTileSets > 0 Then
For x = TileView.Left To TileView.Right
For y = TileView.top To TileView.Bottom
If IsValidMapPoint(x, y) Then
Call BltMapFringeTile(x, y)
End If
Next
Next
End If
' blit out a square at mouse cursor
If InMapEditor Then
If frmEditor_Map.optBlock.Value = True Then
For x = TileView.Left To TileView.Right
For y = TileView.top To TileView.Bottom
If IsValidMapPoint(x, y) Then
Call BltDirection(x, y)
End If
Next
Next
End If
Call BltTileOutline
End If
' Render the bars
BltBars
' Blt the target icon
If myTarget > 0 Then
If myTargetType = TARGET_TYPE_PLAYER Then
BltTarget (Player(myTarget).x * 32) + Player(myTarget).XOffset, (Player(myTarget).y * 32) + Player(myTarget).YOffset
ElseIf myTargetType = TARGET_TYPE_NPC Then
BltTarget (MapNpc(myTarget).x * 32) + MapNpc(myTarget).XOffset, (MapNpc(myTarget).y * 32) + MapNpc(myTarget).YOffset
End If
End If
' blt the hover icon
For i = 1 To Player_HighIndex
If IsPlaying(i) Then
If Player(i).Map = Player(MyIndex).Map Then
If CurX = Player(i).x And CurY = Player(i).y Then
If myTargetType = TARGET_TYPE_PLAYER And myTarget = i Then
' dont render lol
Else
BltHover TARGET_TYPE_PLAYER, i, (Player(i).x * 32) + Player(i).XOffset, (Player(i).y * 32) + Player(i).YOffset
End If
End If
End If
End If
Next
For i = 1 To Npc_HighIndex
If MapNpc(i).num > 0 Then
If CurX = MapNpc(i).x And CurY = MapNpc(i).y Then
If myTargetType = TARGET_TYPE_NPC And myTarget = i Then
' dont render lol
Else
BltHover TARGET_TYPE_NPC, i, (MapNpc(i).x * 32) + MapNpc(i).XOffset, (MapNpc(i).y * 32) + MapNpc(i).YOffset
End If
End If
End If
Next
' Lock the backbuffer so we can draw text and names
TexthDC = DDS_BackBuffer.GetDC
' draw FPS
If BFPS Then
Call DrawText(TexthDC, Camera.Right - (Len("FPS: " & GameFPS) * 8), Camera.top + 1, Trim$("FPS: " & GameFPS), QBColor(Yellow))
End If
' draw cursor, player X and Y locations
If BLoc Then
Call DrawText(TexthDC, Camera.Left, Camera.top + 1, Trim$("cur x: " & CurX & " y: " & CurY), QBColor(Yellow))
Call DrawText(TexthDC, Camera.Left, Camera.top + 15, Trim$("loc x: " & GetPlayerX(MyIndex) & " y: " & GetPlayerY(MyIndex)), QBColor(Yellow))
Call DrawText(TexthDC, Camera.Left, Camera.top + 27, Trim$(" (map #" & GetPlayerMap(MyIndex) & ")"), QBColor(Yellow))
End If
' draw player names
For i = 1 To Player_HighIndex
If IsPlaying(i) And GetPlayerMap(i) = GetPlayerMap(MyIndex) Then
Call DrawPlayerName(i)
End If
Next
' draw npc names
For i = 1 To Npc_HighIndex
If MapNpc(i).num > 0 Then
Call DrawNpcName(i)
End If
Next
For i = 1 To Action_HighIndex
Call BltActionMsg(i)
Next i
' Blit out map attributes
If InMapEditor Then
Call BltMapAttributes
End If
' Draw map name
Call DrawText(TexthDC, DrawMapNameX, DrawMapNameY, Map.Name, DrawMapNameColor)
' Release DC
DDS_BackBuffer.ReleaseDC TexthDC
' Get rec
With rec
.top = Camera.top
.Bottom = .top + ScreenY
.Left = Camera.Left
.Right = .Left + ScreenX
End With
' rec_pos
With rec_pos
.Bottom = ((MAX_MAPY + 1) * PIC_Y)
.Right = ((MAX_MAPX + 1) * PIC_X)
End With
' Flip and render
DX7.GetWindowRect frmMain.picScreen.hWnd, rec_pos
DDS_Primary.Blt rec_pos, DDS_BackBuffer, rec, DDBLT_WAIT
' Error handler
Exit Sub
errorhandler:
HandleError "Render_Graphics", "modDirectDraw7", Err.Number, Err.Description, Err.Source, Err.HelpContext
Err.Clear
Exit Sub
End Sub
``` -
I would like to BLT things to the picScreen,
i had tried this tutorial:
http://www.touchofdeathforums.com/smf2/index.php/topic,79624.0
but it doesn't work,
no error but it just wouldn't appear… any idea?
(i created a .bmp with pink background and a icon on it,
and placed it in the right folder) -
if no one want it, ok… nevermind, topic close :)
-
for some people, it is good offer :)
-
anyway why so many people are watching but no people want to apply?
-
not many people are able to afford server, include me, if you want a server for test, i can apply one for you, and you can try the quality :)
anyway you are the main developer of EO~ -
Robin:
linux is cheaper than windows server, anyway this is free~
Kamii:
Yes! Every server have 256MB swap! isn't good? but anyway RAM is for limited time(swap) so better dun use much swap RAM -
after opened the eclipse server… it only left about 10mb of RAM!
running DE (Desktop environment) in 128mb RAM (Linux) is almost impossible! people atleast need 256mb to done that
i just done a test:
Debian OS - 37MB RAM
VNC - 16MB RAM
Desktop GUI - 4MB RAM
Terminal - 2MB RAM
Wine + Eclipse Server - 55MB RAM
**Left - 13MB RAM**
so do you think it can allow so many player?
**EDIT: Sorry, i had try once more, it work very fine, about 4 player = 1MB RAM
so… this can host 52 player (eclipse origins 2.0)
other server may have more item or player, so best recommended is 30 player** -
This server is tested only MAX 50* players to connect with, recommend 30 players
* Based on Fresh Eclipse Origin
I will help anyone who need to host their server, actually the server is not mine, it is provided by a company, i have the way to make it free forever :D, but it is not illegal, the people who apply to will have the root control and remote desktop (just like RDP but it is VNC)
**The server spec:**
RAM: 128MB
OS: Debian 5 Lenny
Disk Space: 2.5GB (Actual free space: 1.5GB (After minus the OS, Wine, EO Runtimes)
Eclipse server is work in Wine, Wine is a program for linux to run windows program in linux
The only bad thing is the ip will be changed every few days so i will assign into dyndns so you all can connect to it easily
Apply example:
> DynDNS Address: freesmallserver.dyndns.org
> Game name: Doraemon
After i had replied or private messaged to you, you will needed to send me the information of your server (server password & the zip file of your eclipse server)
You can change your server password by typing "passwd" on Putty (Go google search putty, it is used to control your server via terminal)
To prevent abuse, the people who apply must have atleast 50 post in eclipse forum and it is only limited to 10 people. Currently…
Server left: **10/10!** -
vbforums is a very good place to ask :)
-
FULL LIST - Tested by Me
| **Feature** | **0-Player** | **1-Moderator** | **2-Mapper** | **3-Developer** | **4-Owner** |
| Kick | No | No* | No* | No* | No* |
| Ban | No | No | Yes | Yes | Yes |
| Warp2Me | No | No | Yes | Yes | Yes |
| WarpMe2 | No | No | Yes | Yes | Yes |
| Set Access | No | No | No | No | Yes |
| Warp To Map | No | No | Yes | Yes | Yes |
| Set Sprite | No | No | Yes | Yes | Yes |
| Map | No | No | Yes | Yes | Yes |
| Item | No | No | No | Yes | Yes |
| Resource | No | No | No | Yes | Yes |
| NPC | No | No | No | Yes | Yes |
| Spell | No | No | No | Yes | Yes |
| Shop | No | No | No | Yes | Yes |
| Animation | No | No | No | Yes | Yes |
| Loc | No | No | Yes | Yes | Yes |
| Map Report | No | No | Yes | Yes | Yes |
| Del Bans | No | No | No | No | Yes |
| Respawn | No | No | Yes | Yes | Yes |
| Spawn Item | No | No | No | No | Yes |
| Level Up | No | No | No | Yes | Yes |
| Screenshot Map | No | No | Yes | Yes | Yes |
| Colour | Orange | Grey | Aqua Blue | Green | Yellow |
* Not sure if /kick command can or not. -
nvm… thread close ==
-
POST REMOVED
-
THREAD CLOSED FOR A FEW REASON
-
i always love rithy58 reply, it is very useful and long
-
1\. How to make the fog work?
2\. If i add some EO2.0 's feature in it, will it work?
3\. How to make insert own font into the engine… what does "dat" file means? i know "png" but no "dat"
4\. How can i let EO's character work on CS:DE? can we change the code?
For example:
EO is
█░█░
░█░█
█░█░
░█░█
but CS:DE is
█░█
░█░
█░█
░█░ -
Robin is so good… a few years ago, he provides us the eclipse stable, then evolution, then now origins, he does these for what? Does he get some advantage? It is almost 0 advantage, now he still provides us his history bundle... but what he get? Nothing! Robin let some people that basic in VB who need to learn about creating Online Game a engine. Such that good people, it is hard to find in the world, go see other site, MMORPG maker, if u want a good, better engine? MONEY... Robin is also very humor when talking. So i think Robin will be a successfully man in future.
Please share your comment too -
i had successfully made it but… aw... i just cant make it work on linux server via wine bcuz i have a linux server... windows server are too expensive...
-
basiclly u would no need tat high of disk space for EO client, if u want an server with 10GB HDD, 512MB RAM(Burst), 500GB BW, it is just $36 per year!, or you prefer bigger space: 20GB HDD, 1GB RAM(Burst), 1TB BW and it is $72 per year… just buy it from http://123systems.net it is cheap... u can check out my tutorial for setting it for working with EO server: http://www.touchofdeathforums.com/smf/index.php/topic,73451.msg787733.html#msg787733
-
buy, not register ==
-
it should be at here:
Server.vbp -> modConstants (It is at the right top) -> Scroll down until u see this:
' Default starting location [Server Only]
Public Const START_MAP As Long = 1
Public Const START_X As Long = 1
Public Const START_Y As Long = 1
Just change these value… -
just describe… i try to understand...
-
bump… anyone can help me? robin... mr sean... or kamii... atleast give me some tips on it?
How to BLT something to picScreen?
in Q & A
Posted
I tried that before but it doesn't work because it is rendered before the ground's tile… :p