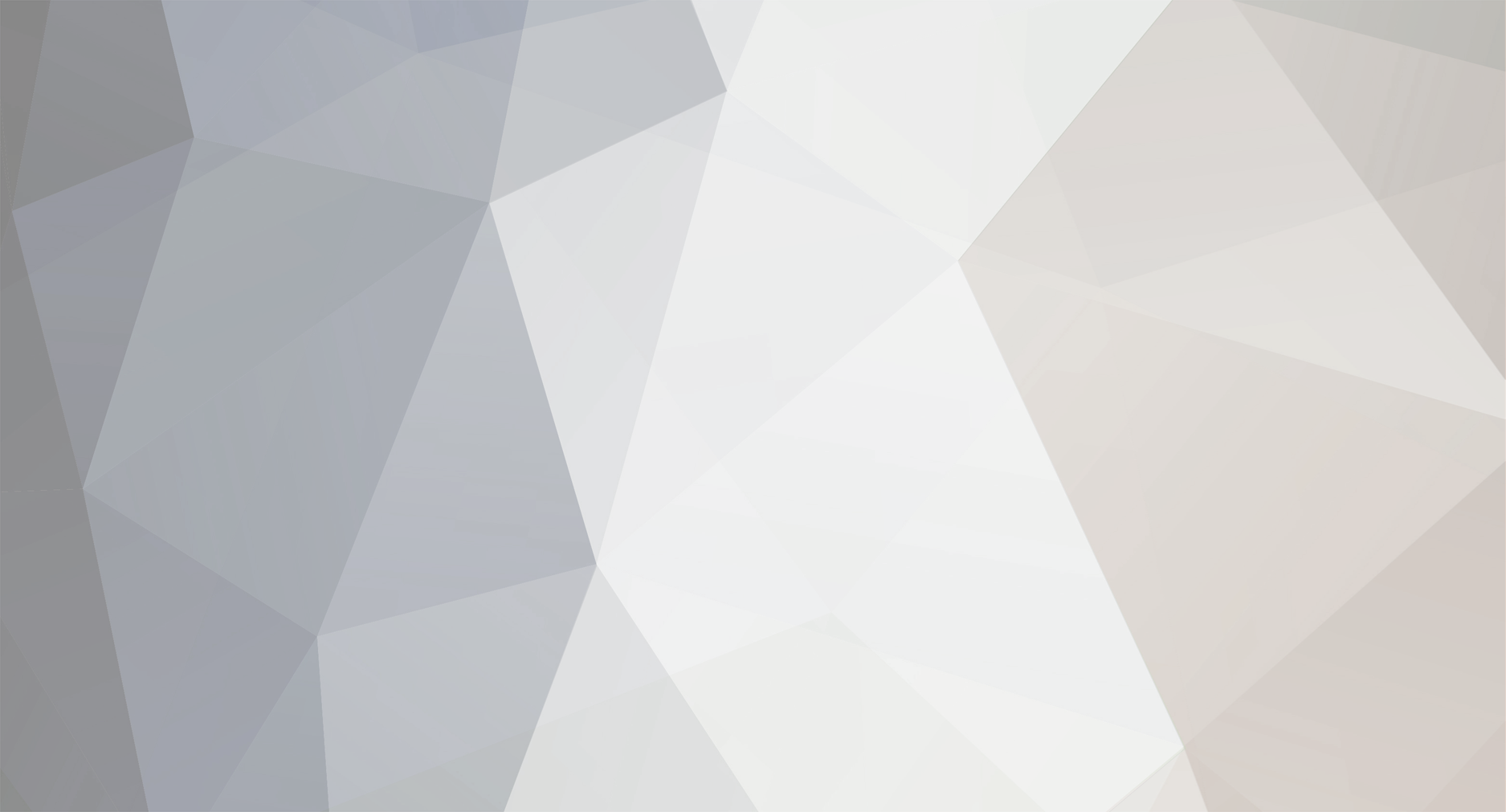
viciousdead
-
Posts
990 -
Joined
-
Last visited
Never
Content Type
Profiles
Forums
Calendar
Posts posted by viciousdead
-
-
Okay, confused here.. Does it end or start 9/4/12?
-
Sorry about inactivity, I PROMISE will get to the animations.
-
@Sealbreaker:
> ```
> // Priority Level
> Thread.currentThread().setPriority(Thread.MAX_PRIORITY); // why do you do this?
>
> ```
I'm not really sure, I was told it was neccesary for the thread to run(by a java forum), but I just removed it and it worked fine… I'll try to add fps, thanks for the help. -
Posting it, but I would like if no one made fun of my horrid coding skills or my random //descriptions. <3
*You need to resize the applet via HTML.
Player(Sub, Stores Player Variables):
```
import java.awt.*;
public class Player
{
public int radius = 50; // Circle Size
public int x = 50; // X Value
public int y = 90; // Y Value
public int spd = 10; // Speed Of Circle
public double hp = 2000; // Health Value
// Special Damage And Range
public int sp1_range = 150; // Special One's Range
public double sp1_dmg = 1.5; // Special One's Damage
public boolean space; // Pressed Or Not
public boolean left; // Pressed Or Not
public boolean right; // Pressed Or Not
public boolean up; // Pressed Or Not
public boolean down; // Pressed Or Not
}
```
Mob1(Sub, Stores AI Variables):
```
import java.awt.*;
public class Mob1
{
public int radius = 30; // Circle Size
public int x = 550; // X Value
public int y = 550; // Y Value
public int spd = 2; // Speed Of Circle
public double hp = 1000; // Health Value
public boolean space; // Pressed Or Not
public boolean left; // Pressed Or Not
public boolean right; // Pressed Or Not
public boolean up; // Pressed Or Not
public boolean down; // Pressed Or Not
}
```
GridKeyListener(KeyEventHandler, ignore the name from an old grid thing I was doing..):
```
import java.awt.event.*;
public class GridKeyListener implements KeyListener
{
boolean space = false;
boolean left = false;
boolean right = false;
boolean up = false;
boolean down = false;
public void keyPressed(KeyEvent e)
{
switch (e.getKeyCode())
{
case KeyEvent.VK_SPACE:
space = true;
break;
case KeyEvent.VK_LEFT:
left = true;
break;
case KeyEvent.VK_RIGHT:
right = true;
break;
case KeyEvent.VK_UP:
up = true;
break;
case KeyEvent.VK_DOWN:
down = true;
}
}
public void keyReleased(KeyEvent e)
{
switch (e.getKeyCode())
{
case KeyEvent.VK_SPACE:
space = false;
break;
case KeyEvent.VK_LEFT:
left = false;
break;
case KeyEvent.VK_RIGHT:
right = false;
break;
case KeyEvent.VK_UP:
up = false;
break;
case KeyEvent.VK_DOWN:
down = false;
}
}
public void keyTyped(KeyEvent e){}
public boolean getSpace(){return space;}
public boolean getLeft(){return left;}
public boolean getRight(){return right;}
public boolean getUp(){return up;}
public boolean getDown(){return down;}
}
```
Game(Main, basically everything is done here..):
```
import java.applet.*;
import java.awt.*;
public class Game extends Applet implements Runnable
{
// Double Buffer
Graphics bufferGraphics;
Image offscreen;
Dimension dim;
// Creates Communication Between Other Classes
public GridKeyListener gkl = new GridKeyListener();
public Player p1 = new Player();
public Mob1 m1 = new Mob1();
// Define Images
private Image spaceAtk;
// First Operation
public void init()
{
// Sets Background Color
setBackground (Color.black);
// Double Buffer
dim = getSize();
offscreen = createImage(dim.width,dim.height);
bufferGraphics = offscreen.getGraphics();
}
// Start The Program
public void start()
{
// Defines And Starts Thread; Adds KeyListener
Thread th = new Thread (this);
this.addKeyListener(gkl);
th.start ();
}
public void stop(){}
public void destroy(){}
public void run()
{
// Priority Level
Thread.currentThread().setPriority(Thread.MIN_PRIORITY);
while (true)
{
// Refresh
repaint();
try
{
// Stops Thread For 20 Milliseconds
Thread.sleep (20);
}
catch (InterruptedException ex)
{
}
// Priority Level
Thread.currentThread().setPriority(Thread.MAX_PRIORITY);
}
}
public void paint (Graphics g)
{
// Double Buffer
bufferGraphics.clearRect(0,0,dim.width,dim.width);
// Load Pictures
spaceAtk = getImage(getCodeBase(), "test.png");
// Check Directions - Player
p1.space = gkl.getSpace();
p1.left = gkl.getLeft();
p1.right = gkl.getRight();
p1.up = gkl.getUp();
p1.down = gkl.getDown();
// Check Directions - AI
if(m1.x > p1.x){m1.left = true;} else {m1.left = false;}
if(m1.x < p1.x){m1.right = true;} else {m1.right = false;}
if(m1.y > p1.y){m1.up = true;} else {m1.up = false;}
if(m1.y < p1.y){m1.down = true;} else {m1.down = false;}
// Boundaries - Player
if(p1.y <= 90){p1.up = false;}
if(p1.y >= 600){p1.down = false;}
if(p1.x <= 50){p1.left = false;}
if(p1.x >= 900){p1.right = false;}
// Boundaries - AI
if(m1.y <= 90){m1.up = false;}
if(m1.y >= 600){m1.down = false;}
if(m1.x <= 50){m1.left = false;}
if(m1.x >= 900){m1.right = false;}
// Platforms - Player
if(p1.x <= 750 && p1.y == 190 - p1.radius){p1.down = false;}
if(p1.x <= 750 && p1.y == 200){p1.up = false;}
if(p1.x >= 150 && p1.y == 290 - p1.radius){p1.down = false;}
if(p1.x >= 150 && p1.y == 300){p1.up = false;}
// Platforms - AI
if(m1.x <= 750 && m1.y == 190 - m1.radius){m1.down = false;}
if(m1.x <= 750 && m1.y == 200){m1.up = false;}
if(m1.x >= 150 && m1.y == 290 - m1.radius){m1.down = false;}
if(m1.x >= 150 && m1.y == 300){m1.up = false;}
// Draws Circle
if(p1.hp>0)
{
bufferGraphics.setColor (Color.red);
bufferGraphics.fillOval (p1.x - p1.radius, p1.y - p1.radius, 2 * p1.radius, 2 * p1.radius);
}
if(m1.hp>0)
{
bufferGraphics.setColor (Color.green);
bufferGraphics.fillOval (m1.x - m1.radius, m1.y - m1.radius, 2 * m1.radius, 2 * m1.radius);
}
// Movement - Player
if(p1.left){p1.x -= p1.spd;}
if(p1.right){p1.x += p1.spd;}
if(p1.up){p1.y -= p1.spd;}
if(p1.down){p1.y += p1.spd;}
// Movement - AI
if(m1.left){m1.x -= m1.spd;}
if(m1.right){m1.x += m1.spd;}
if(m1.up){m1.y -= m1.spd;}
if(m1.down){m1.y += m1.spd;}
// Special Attacks
if(p1.hp>0 && p1.space){bufferGraphics.drawImage (spaceAtk, p1.x-2 * p1.radius, p1.y - 2 * p1.radius, this);}
// Damage Code
if(p1.hp>0 && (m1.x - m1.radius <= p1.x + p1.sp1_range) && (m1.y - m1.radius <= p1.y + p1.sp1_range) && (m1.x + m1.radius >= p1.x - p1.sp1_range) && (m1.y + m1.radius >= p1.y - p1.sp1_range)){
if(p1.space){
m1.hp -= p1.sp1_dmg;
}
}
if((m1.hp > 0) && (p1.x - p1.radius <= m1.x) && (p1.y - p1.radius <= m1.y) && (p1.x + p1.radius >= m1.x) && (p1.y + p1.radius >= m1.y)){
p1.hp -= 0.5;
}
// Fix Negative Health
if(p1.hp<0){p1.hp = 0;}
if(m1.hp<0){m1.hp = 0;}
// Draws Text
String s_msg = "Space: " + p1.space;
String r_msg = "Right: " + p1.right;
String l_msg = "Left: " + p1.left;
String u_msg = "Up: " + p1.up;
String d_msg = "Down: " + p1.down;
String hp_msg = "Health: " + p1.hp;
String ehp_msg = "Enemy Health: " + m1.hp;
String up_msg = "^";
String down_msg = "v";
String left_msg = "<";
String right_msg = ">";
Font small = new Font("Helvetica", Font.BOLD, 32);
FontMetrics metr = this.getFontMetrics(small);
bufferGraphics.setColor(Color.cyan);
bufferGraphics.setFont(small);
bufferGraphics.drawString(hp_msg, (290 - metr.stringWidth(hp_msg)), 500);
bufferGraphics.drawString(ehp_msg, (400 - metr.stringWidth(ehp_msg)), 560);
bufferGraphics.drawString(s_msg, (260 - metr.stringWidth(s_msg)), 620);
bufferGraphics.setColor(Color.white);
bufferGraphics.drawString(right_msg, (100 - metr.stringWidth(right_msg)), 80);
bufferGraphics.drawString(right_msg, (300 - metr.stringWidth(right_msg)), 80);
bufferGraphics.drawString(right_msg, (500 - metr.stringWidth(right_msg)), 80);
bufferGraphics.drawString(right_msg, (700 - metr.stringWidth(right_msg)), 80);
bufferGraphics.drawString(down_msg, (900 - metr.stringWidth(down_msg)), 80);
bufferGraphics.drawString(left_msg, (900 - metr.stringWidth(left_msg)), 240);
bufferGraphics.drawString(left_msg, (700 - metr.stringWidth(left_msg)), 240);
bufferGraphics.drawString(left_msg, (500 - metr.stringWidth(left_msg)), 240);
bufferGraphics.drawString(left_msg, (300 - metr.stringWidth(left_msg)), 240);
bufferGraphics.drawString(down_msg, (100 - metr.stringWidth(down_msg)), 240);
// Draw Entire Double Buffered Image
g.drawImage(offscreen,0,0,this);
}
public void update(Graphics g)
{
paint(g);
}
}
``` -
-
I've been waiting for this since like 09 or 08.. Can't wait to play… :sad:
-
@Sick:
> Greetings Eclipse! Im pretty sure most of you know the game "Haven and Hearth". And some of you might know their new project, "Salem". It's basicly a 3D version of HH.
>
> Anyways, beta is approaching and I thought I'd share it with you.
>
> Link to beta sign-up: http://beta.salemthegame.com/?ref=e0b84333372
>
> *When Beta is open, feel free to discuss it here.*
Signed up, now I want referals… ):
http://beta.salemthegame.com/?ref=7c0f36ee749 -
Did you port forward the ip and port numbers?
-
@LordKronos:
> I dont know where else to go and i need the help….
>
> i saved it as a bmp because thats how the others are saved...
BMP -> loads slow, big file
PNG -> loads fast, small file
Use BMP for your game, but don't post BMP on the forums, instead use PNG. It's to reduce lag and some people can't see BMP(I don't know why).
Scythe looks okay, but it does need more contrasting/defined colors. -
@Pete:
> "Instead of Husbands, they could be… Butt Buddies."
This. ^ :D -
@Sealbreaker:
> hm… i always used a graphics2d object with bufferedimages, it isn't hard at all and you can do some shit with it (rotation, etc.)
> anyhow, for double-buffering you just have a bufferedimage (or whatever image-type you are using) with the size of the screen that you draw on instead of directly drawing to the screen. Then, in your repaint-method you just draw that 1 image which contains all the stuff you've drawn to it... You can look up some tutorials, shoulnd't be too hard to figure that out.
>
> -seal
Okay, I'll do that. Thanks.
EDIT:Double buffering makes it laggy(It's redrawing entire screen, off screen, constantly).. No idea how to reduce lag except make it smaller, lol.. ;) -
@Sealbreaker:
> are you using java2d?
I'm using Graphics, I assume that's the question, otherwise not really sure what you mean by java2D. Do I need to use Graphics2D? -
@Aáron:
> Double Buffer will make it look so much better;)
I know, but I'm to stupid to figure double buffer out. ;D -
@Sealbreaker:
> Didn't see that this was in off-topic…Well then forget what I said because everyone posts random shit in here :P
> The troll image was fun. haha!
Working on adding more balls with forever alone face, lol… -
@Sealbreaker:
> Why would you post something like this to the public?? It's like posting "Look! I learned how to calculate 1+1 !!!" What should we say about something that isn't a serious attempt in any direction?
>
> -seal
Whatever you feel like saying. o.o
EDIT: And you are right, I did learn 1+1! :D -
LMAO THIS SHIT IS BANANAS.
Inb4IWentThere -
Nothing big, just bored. s:
http://justinandjava.vacau.com/Gamez.html
Arrow Keys - movement
Space - attack
Update 1:
Changed AI Movements
Double Buffering + Extreme Lag… Yay! :D
*Requires Java To Run… -
@Crest:
> just examples of their conditions:
>
> >! 
> 
Drools at Samus.
No idea what they're worth, but I'd say at least a hundred for the nes ones. -
@Niall:
> Right, so I quickly opened up the VB project files again and added a little feature, just to help me get back into the swing of things with OTLR.
>
> I've made it so players can have different movement speeds to each other, which also comes with a handy check to get rid of the movement speed exploit. It also makes PvP combat a little more interesting, so players can't simply run away from a fight - unless they are faster than the attacker of course!
>
> I should be posting other updates in the next few weeks - hopefully ones which contain a preliminary tileset/tiles of some sort.
>
> Regards,
>
> Niall
Good to hear, I'll have some new animations to you soon. -
Lol… :confused:
http://www.youtube.com/watch?v=0PAJNntoRgA -
Not trying to insult you or anything, but I could swear that sprite looks like Cro from mini-fighters… ;)
Face looks hand drawn(attribute of Mini-fighters), while the hair and body look pixeled...
Change the face or the entire body, all I can say. -
@Zonova:
> what on earth happened to this thread?
Trolls. -
@Robin:
> Woah there tiger. Calm down. We're insulting the education system, not your work.
>
> We're teasing you because you're bragging about a God damn school project. Keep in mind that forum interactions are just the same in real life.
>
> How would you think your friends would react if you went to a house party with a print out of your school report going on about what you're doing with your tutor.
>
> You need some thicker skin.
Well I should've kept it simple, and just posted the finished product. Maybe I wouldn't have been trolled/started a debate about school systems. Yes, I need thicker skin. /= -
@mig:
> Thanks.
>
> 
Lol, Ily. So why did you make this thread? xD
[WIP] Aegis
in Old Eclipse Projects (Inactive)
Posted
> Generally, MM/DD/YY is used in America and DD/MM/YY is used in Europe.
Lol, thanks for the info, thought he meant it started September..